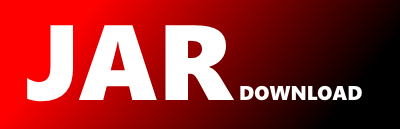
com.metaeffekt.artifact.enrichment.details.DetailsFillingEnrichment Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.details;
import com.metaeffekt.artifact.enrichment.InventoryEnricher;
import com.metaeffekt.artifact.enrichment.configurations.details.DetailsFillingInventoryEnrichmentConfiguration;
import com.metaeffekt.mirror.contents.advisory.AdvisoryEntry;
import com.metaeffekt.mirror.contents.base.VulnerabilityContextInventory;
import com.metaeffekt.mirror.contents.vulnerability.Vulnerability;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Supplier;
public abstract class DetailsFillingEnrichment> extends InventoryEnricher {
private static final Logger LOG = LoggerFactory.getLogger(DetailsFillingEnrichment.class);
protected C configuration;
@Override
public C getConfiguration() {
return configuration;
}
public void setConfiguration(C configuration) {
this.configuration = configuration;
}
@Override
protected void performEnrichment(Inventory inventory) {
final VulnerabilityContextInventory vInventory = VulnerabilityContextInventory.fromInventory(inventory);
final AtomicInteger appliedVulnerabilities = new AtomicInteger(0);
final AtomicInteger appliedAdvisories = new AtomicInteger(0);
super.executor.setSize(16);
for (Vulnerability vulnerability : vInventory.getShallowCopyVulnerabilities()) {
if (isApplicable(vulnerability)) {
appliedVulnerabilities.incrementAndGet();
super.executor.submit(() -> fillDetailsOnVulnerability(inventory, vInventory, vulnerability));
}
}
LOG.info("Starting vulnerability details filling on [{} / {}] vulnerabilities", appliedVulnerabilities.get(), vInventory.getVulnerabilities().size());
super.executor.start();
try {
executor.join();
} catch (InterruptedException e) {
throw new RuntimeException("Failed to wait for vulnerability details filling.", e);
}
for (AdvisoryEntry advisory : vInventory.getShallowCopySecurityAdvisories()) {
if (isApplicable(advisory)) {
appliedAdvisories.incrementAndGet();
super.executor.submit(() -> fillDetailsOnAdvisory(inventory, vInventory, advisory));
}
}
LOG.info("Starting advisory details filling on [{} / {}] advisories", appliedAdvisories.get(), vInventory.getSecurityAdvisories().size());
super.executor.start();
try {
executor.join();
} catch (InterruptedException e) {
throw new RuntimeException("Failed to wait for vulnerability details filling.", e);
}
vInventory.writeBack(true);
}
protected abstract boolean isApplicable(Vulnerability vulnerability);
protected abstract boolean isApplicable(AdvisoryEntry advisory);
protected abstract void fillDetailsOnVulnerability(Inventory inventory, VulnerabilityContextInventory vInventory, Vulnerability queryVulnerability);
protected abstract void fillDetailsOnAdvisory(Inventory inventory, VulnerabilityContextInventory vInventory, AdvisoryEntry queryAdvisory);
protected List pickNonEmptyQueryResult(Supplier selfInstanceSupplier, Supplier> otherInstancesSupplier) {
final T selfInstance = selfInstanceSupplier.get();
if (selfInstance != null) {
return Collections.singletonList(selfInstance);
}
final List otherInstances = otherInstancesSupplier.get();
if (otherInstances == null || otherInstances.isEmpty()) {
return Collections.emptyList();
}
return otherInstances;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy