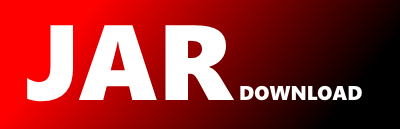
com.metaeffekt.artifact.enrichment.details.DetailsFillingEnrichmentMsrc Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.details;
import com.metaeffekt.artifact.analysis.utils.LazySupplier;
import com.metaeffekt.artifact.analysis.utils.StringUtils;
import com.metaeffekt.artifact.analysis.vulnerability.enrichment.InventoryAttribute;
import com.metaeffekt.artifact.enrichment.configurations.details.DetailsFillingMsrcInventoryEnrichmentConfiguration;
import com.metaeffekt.mirror.contents.advisory.AdvisoryEntry;
import com.metaeffekt.mirror.contents.advisory.MsrcAdvisorEntry;
import com.metaeffekt.mirror.contents.base.DataSourceIndicator;
import com.metaeffekt.mirror.contents.base.VulnerabilityContextInventory;
import com.metaeffekt.mirror.contents.msrcdata.MsrcSupersedeNode;
import com.metaeffekt.mirror.contents.store.AdvisoryTypeStore;
import com.metaeffekt.mirror.contents.store.VulnerabilityTypeStore;
import com.metaeffekt.mirror.contents.vulnerability.Vulnerability;
import com.metaeffekt.mirror.download.documentation.EnricherMetadata;
import com.metaeffekt.mirror.download.documentation.InventoryEnrichmentPhase;
import com.metaeffekt.mirror.query.MsrcAdvisorIndexQuery;
import com.metaeffekt.mirror.query.MsrcKbChainIndexQuery;
import org.json.JSONArray;
import org.json.JSONObject;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
@EnricherMetadata(
name = "MSRC Vulnerability Details", phase = InventoryEnrichmentPhase.VULNERABILITY_DETAILS_FILLING,
intermediateFileSuffix = "details-vulnerability-msrc", mavenPropertyName = "msrcAdvisorFillDetailsEnrichment",
explicitConfiguration = DetailsFillingMsrcInventoryEnrichmentConfiguration.class
)
public class DetailsFillingEnrichmentMsrc extends DetailsFillingEnrichment {
private static final Logger LOG = LoggerFactory.getLogger(DetailsFillingEnrichmentMsrc.class);
private final LazySupplier msrcAdvisorQuery;
private final LazySupplier msrcKbChainIndexQuery;
public DetailsFillingEnrichmentMsrc(File baseMirrorDirectory) {
this.msrcAdvisorQuery = new LazySupplier<>(() -> new MsrcAdvisorIndexQuery(baseMirrorDirectory));
this.msrcKbChainIndexQuery = new LazySupplier<>(() -> new MsrcKbChainIndexQuery(baseMirrorDirectory));
setConfiguration(new DetailsFillingMsrcInventoryEnrichmentConfiguration());
}
@Override
protected boolean isApplicable(Vulnerability vulnerability) {
final String name = vulnerability.getId();
if (!AdvisoryTypeStore.MSRC.patternMatchesId(name) && !VulnerabilityTypeStore.CVE.patternMatchesId(name)) {
return false;
}
return true;
}
@Override
protected boolean isApplicable(AdvisoryEntry advisory) {
return advisory.getSourceIdentifier() == AdvisoryTypeStore.MSRC && StringUtils.isEmpty(advisory.getSummary()) && advisory.getDescription().isEmpty();
}
@Override
protected void fillDetailsOnVulnerability(Inventory inventory, VulnerabilityContextInventory vInventory, Vulnerability queryVulnerability) {
final List advisorEntries = super.pickNonEmptyQueryResult(
() -> msrcAdvisorQuery.get().findById(queryVulnerability.getId()),
() -> msrcAdvisorQuery.get().findByReferencedId(queryVulnerability.getId())
);
if (advisorEntries.isEmpty()) {
return;
}
for (MsrcAdvisorEntry advisoryEntry : advisorEntries) {
final AdvisoryEntry constructedAdvisory = vInventory.findOrCreateAdvisoryEntryByName(advisoryEntry.getId(), MsrcAdvisorEntry::new);
constructedAdvisory.addMatchingSource(DataSourceIndicator.vulnerability(queryVulnerability));
queryVulnerability.addSecurityAdvisory(constructedAdvisory);
if (isApplicable(constructedAdvisory)) {
fillDetailsOnAdvisory(inventory, vInventory, constructedAdvisory);
}
}
final Set affectedArtifacts = queryVulnerability.getAffectedArtifactsByDefaultKey();
if (affectedArtifacts == null) return;
final Set msrcProductIdsOnArtifacts = MsrcAdvisorEntry.getAllMsrcProductIds(affectedArtifacts);
final String msFixingIdValue = queryVulnerability.getAdditionalAttribute(InventoryAttribute.MS_FIXING_KB_IDENTIFIER);
final JSONObject fixingKbIds = StringUtils.hasText(msFixingIdValue) ? new JSONObject(msFixingIdValue) : new JSONObject();
for (MsrcAdvisorEntry advisoryEntry : queryVulnerability.getRelatedAdvisors(AdvisoryTypeStore.MSRC, MsrcAdvisorEntry.class)) {
for (String msrcProductIdsOnRelatedArtifact : msrcProductIdsOnArtifacts) {
if (advisoryEntry.getAffectedProducts().contains(msrcProductIdsOnRelatedArtifact)) {
final Set kbIds = msrcKbChainIndexQuery.get().findFixingKbIds(queryVulnerability.getId(), msrcProductIdsOnRelatedArtifact);
if (kbIds.isEmpty()) continue;
final JSONArray fixingKbIdsForProduct = fixingKbIds.optJSONArray(msrcProductIdsOnRelatedArtifact) == null ? new JSONArray() : fixingKbIds.getJSONArray(msrcProductIdsOnRelatedArtifact);
fixingKbIds.put(msrcProductIdsOnRelatedArtifact, fixingKbIdsForProduct);
for (MsrcSupersedeNode kbId : kbIds) {
fixingKbIdsForProduct.put(kbId.getKbId());
}
}
}
}
if (!fixingKbIds.isEmpty()) {
// make all distinct
fixingKbIds.keySet().forEach(key -> {
final JSONArray fixingKbIdsForProduct = fixingKbIds.getJSONArray(key);
final Set distinctKbIds = fixingKbIdsForProduct.toList().stream().map(Object::toString).collect(Collectors.toSet());
fixingKbIds.put(key, distinctKbIds);
});
queryVulnerability.setAdditionalAttribute(InventoryAttribute.MS_FIXING_KB_IDENTIFIER, fixingKbIds.toString());
}
}
@Override
protected void fillDetailsOnAdvisory(Inventory inventory, VulnerabilityContextInventory vInventory, AdvisoryEntry queryAdvisory) {
final MsrcAdvisorEntry foundAdvisory = msrcAdvisorQuery.get().findById(queryAdvisory.getId());
if (foundAdvisory != null) {
queryAdvisory.appendFromDataClass(foundAdvisory);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy