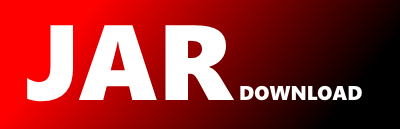
com.metaeffekt.artifact.enrichment.details.DetailsFillingEnrichmentNvd Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.details;
import com.metaeffekt.artifact.analysis.utils.LazySupplier;
import com.metaeffekt.artifact.analysis.utils.StringUtils;
import com.metaeffekt.artifact.enrichment.configurations.details.DetailsFillingNvdInventoryEnrichmentConfiguration;
import com.metaeffekt.mirror.contents.advisory.AdvisoryEntry;
import com.metaeffekt.mirror.contents.base.VulnerabilityContextInventory;
import com.metaeffekt.mirror.contents.store.VulnerabilityTypeStore;
import com.metaeffekt.mirror.contents.vulnerability.Vulnerability;
import com.metaeffekt.mirror.download.documentation.EnricherMetadata;
import com.metaeffekt.mirror.download.documentation.InventoryEnrichmentPhase;
import com.metaeffekt.mirror.query.NvdCveIndexQuery;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.Optional;
@EnricherMetadata(
name = "NVD Vulnerability Details", phase = InventoryEnrichmentPhase.VULNERABILITY_DETAILS_FILLING,
intermediateFileSuffix = "details-vulnerability-nvd", mavenPropertyName = "nvdCveFillDetailsEnrichment",
explicitConfiguration = DetailsFillingNvdInventoryEnrichmentConfiguration.class
)
public class DetailsFillingEnrichmentNvd extends DetailsFillingEnrichment {
private static final Logger LOG = LoggerFactory.getLogger(DetailsFillingEnrichmentNvd.class);
private final LazySupplier nvdQuery;
private DetailsFillingNvdInventoryEnrichmentConfiguration configuration = new DetailsFillingNvdInventoryEnrichmentConfiguration();
public DetailsFillingEnrichmentNvd(File baseMirrorDirectory) {
nvdQuery = new LazySupplier<>(() -> new NvdCveIndexQuery(baseMirrorDirectory));
}
public void setConfiguration(DetailsFillingNvdInventoryEnrichmentConfiguration configuration) {
this.configuration = configuration;
}
@Override
public DetailsFillingNvdInventoryEnrichmentConfiguration getConfiguration() {
return configuration;
}
@Override
public boolean isApplicable(Vulnerability vulnerability) {
return StringUtils.isEmpty(vulnerability.getDescription())
&& VulnerabilityTypeStore.CVE == vulnerability.getSourceIdentifier();
}
@Override
protected boolean isApplicable(AdvisoryEntry advisory) {
// does not provide advisories
return false;
}
@Override
protected void fillDetailsOnVulnerability(Inventory inventory, VulnerabilityContextInventory vInventory, Vulnerability queryVulnerability) {
final Optional potentialDbVulnerability = nvdQuery.get().findVulnerabilityByName(queryVulnerability.getId());
if (!potentialDbVulnerability.isPresent()) {
LOG.warn("CVE-Vulnerability [{}] not found in NVD", queryVulnerability.getId());
} else {
final Vulnerability dbVulnerability = potentialDbVulnerability.get();
queryVulnerability.appendFromDataClass(dbVulnerability);
}
}
@Override
protected void fillDetailsOnAdvisory(Inventory inventory, VulnerabilityContextInventory vInventory, AdvisoryEntry queryAdvisory) {
// NVD does not provide advisories
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy