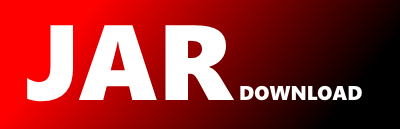
com.metaeffekt.artifact.enrichment.matching.CustomVulnerabilitiesFromCpeEnrichment Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.matching;
import com.metaeffekt.artifact.enrichment.configurations.CustomVulnerabilitiesFromCpeEnrichmentConfiguration;
import com.metaeffekt.mirror.contents.base.VulnerabilityContextInventory;
import com.metaeffekt.mirror.contents.store.ContentIdentifierStore;
import com.metaeffekt.mirror.contents.store.VulnerabilityTypeStore;
import com.metaeffekt.mirror.download.documentation.EnricherMetadata;
import com.metaeffekt.mirror.download.documentation.InventoryEnrichmentPhase;
import com.metaeffekt.mirror.query.CustomVulnerabilityIndexQuery;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.List;
@EnricherMetadata(
name = "Custom Vulnerabilities from CPE", phase = InventoryEnrichmentPhase.VULNERABILITY_MATCHING,
intermediateFileSuffix = "custom-vulnerabilities-from-cpe", mavenPropertyName = "customVulnerabilitiesFromCpeEnrichment"
)
public class CustomVulnerabilitiesFromCpeEnrichment extends VulnerabilitiesFromCpeEnrichment {
private final static Logger LOG = LoggerFactory.getLogger(CustomVulnerabilitiesFromCpeEnrichment.class);
protected CustomVulnerabilityIndexQuery vulnerabilityQuery;
public CustomVulnerabilitiesFromCpeEnrichment(File baseMirrorDirectory) {
super(new CustomVulnerabilitiesFromCpeEnrichmentConfiguration());
}
@Override
public CustomVulnerabilitiesFromCpeEnrichmentConfiguration getConfiguration() {
return (CustomVulnerabilitiesFromCpeEnrichmentConfiguration) super.configuration;
}
@Override
protected CustomVulnerabilityIndexQuery getVulnerabilityQuery() {
return vulnerabilityQuery;
}
@Override
protected ContentIdentifierStore.ContentIdentifier getVulnerabilitySource() {
return VulnerabilityTypeStore.get().fromNameAndImplementationWithoutCreation("CUSTOM", "CUSTOM");
}
@Override
protected void performEnrichment(Inventory inventory) {
vulnerabilityQuery = new CustomVulnerabilityIndexQuery(getConfiguration().getVulnerabilityFiles());
final List artifacts = inventory.getArtifacts();
final VulnerabilityContextInventory vInventory = VulnerabilityContextInventory.fromInventory(inventory);
for (int i = 0, artifactsSize = artifacts.size(); i < artifactsSize; i++) {
final Artifact artifact = artifacts.get(i);
int finalI = i;
super.executor.submit(() -> {
LOG.info("Collecting vulnerabilities from CPE for artifact [{} / {}] [{}: {} {}]", finalI + 1, inventory.getArtifacts().size(), artifact.getId(), artifact.get(Artifact.Attribute.COMPONENT), artifact.get(Artifact.Attribute.VERSION));
super.enrichVulnerabilitiesForCpe(vInventory, artifact);
});
}
super.executor.setSize(16);
super.executor.start();
try {
executor.join();
} catch (InterruptedException e) {
throw new RuntimeException("Interrupted while waiting for custom vulnerability enrichment to finish.", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy