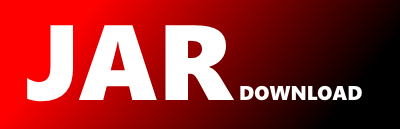
com.metaeffekt.artifact.enrichment.other.timeline.VulnerabilityTimelineGenerator Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.other.timeline;
import com.metaeffekt.artifact.analysis.utils.CountdownTimer;
import com.metaeffekt.artifact.enrichment.configurations.VulnerabilityAssessmentDashboardEnrichmentConfiguration;
import com.metaeffekt.mirror.concurrency.ScheduledDelayedThreadPoolExecutor;
import com.metaeffekt.mirror.contents.vulnerability.Vulnerability;
import com.metaeffekt.mirror.query.NvdCpeApiIndexQuery;
import com.metaeffekt.mirror.query.NvdCveIndexQuery;
import org.apache.commons.lang3.tuple.Pair;
import org.metaeffekt.core.inventory.processor.report.configuration.CentralSecurityPolicyConfiguration;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import us.springett.parsers.cpe.Cpe;
import java.util.*;
public class VulnerabilityTimelineGenerator {
private final static Logger LOG = LoggerFactory.getLogger(VulnerabilityTimelineGenerator.class);
private final Set relevantVulnerabilities = new HashSet<>();
private final Set> generateForVendorProducts = new HashSet<>();
private final NvdCveIndexQuery vulnerabilityQuery;
private final NvdCpeApiIndexQuery cpeDictionaryQuery;
private final VulnerabilityAssessmentDashboardEnrichmentConfiguration vadConfiguration;
private final CentralSecurityPolicyConfiguration centralSecurityPolicyConfiguration;
private final CountdownTimer timelineGenerationTime;
public VulnerabilityTimelineGenerator(NvdCveIndexQuery vulnerabilityQuery, NvdCpeApiIndexQuery cpeDictionaryQuery, VulnerabilityAssessmentDashboardEnrichmentConfiguration vadConfiguration, CentralSecurityPolicyConfiguration centralSecurityPolicyConfiguration) {
this.vulnerabilityQuery = vulnerabilityQuery;
this.cpeDictionaryQuery = cpeDictionaryQuery;
this.vadConfiguration = vadConfiguration;
this.centralSecurityPolicyConfiguration = centralSecurityPolicyConfiguration;
this.timelineGenerationTime = new CountdownTimer(vadConfiguration.getMaximumTimeSpentOnTimelines() * 1000L);
}
public void addRelevantVulnerability(String vulnerabilityId) {
relevantVulnerabilities.add(vulnerabilityId);
}
public void addRelevantVulnerabilities(Collection vulnerabilities) {
for (Vulnerability vulnerability : vulnerabilities) {
addRelevantVulnerability(vulnerability.getId());
}
}
public void removeRelevantVulnerability(String vulnerabilityId) {
relevantVulnerabilities.remove(vulnerabilityId);
}
public void addCpe(Cpe cpe) {
generateForVendorProducts.add(Pair.of(cpe.getVendor(), cpe.getProduct()));
}
public void addCpe(Collection cpe) {
cpe.forEach(this::addCpe);
}
public void addVendorProduct(String vendor, String product) {
generateForVendorProducts.add(Pair.of(vendor, product));
}
public Set getRelevantVulnerabilities() {
return relevantVulnerabilities;
}
public Set> getGenerateForVendorProducts() {
return generateForVendorProducts;
}
public NvdCveIndexQuery getVulnerabilityQuery() {
return vulnerabilityQuery;
}
public NvdCpeApiIndexQuery getCpeDictionaryQuery() {
return cpeDictionaryQuery;
}
public VulnerabilityAssessmentDashboardEnrichmentConfiguration getVadConfiguration() {
return vadConfiguration;
}
public CentralSecurityPolicyConfiguration getCentralSecurityPolicyConfiguration() {
return centralSecurityPolicyConfiguration;
}
public CountdownTimer getTimelineGenerationTime() {
return timelineGenerationTime;
}
public VulnerabilityTimelineGeneratorResult generate() {
LOG.info("Generating [{}] timelines for [{}] relevant vulnerabilities", generateForVendorProducts.size(), relevantVulnerabilities.size());
final List timelines = new ArrayList<>();
for (Pair vendorProduct : generateForVendorProducts) {
if (timelineGenerationTime.isEndReached()) {
LOG.info("Maximum time spent on timeline generation reached. Skipping remaining timelines.");
break;
}
if (vendorProduct.getLeft() != null && vendorProduct.getRight() != null) {
timelines.add(new VulnerabilityTimeline(vendorProduct.getLeft(), vendorProduct.getRight(), this));
}
}
return new VulnerabilityTimelineGeneratorResult(timelines);
}
public VulnerabilityTimelineGeneratorResult generate(ScheduledDelayedThreadPoolExecutor executor) {
LOG.info("Generating [{}] timelines for [{}] relevant vulnerabilities", generateForVendorProducts.size(), relevantVulnerabilities.size());
final List timelines = new ArrayList<>();
for (Pair vendorProduct : generateForVendorProducts) {
executor.submit(() -> {
if (timelineGenerationTime.isEndReached()) {
LOG.info("Maximum time spent on timeline generation reached. Skipping timeline generation for [{}] [{}].", vendorProduct.getLeft(), vendorProduct.getRight());
return;
}
if (vendorProduct.getLeft() != null && vendorProduct.getRight() != null) {
final VulnerabilityTimeline timeline = new VulnerabilityTimeline(vendorProduct.getLeft(), vendorProduct.getRight(), this);
synchronized (timelines) {
timelines.add(timeline);
}
}
});
}
executor.start();
try {
executor.join();
} catch (InterruptedException e) {
throw new RuntimeException("Thread execution interrupted.", e);
}
return new VulnerabilityTimelineGeneratorResult(timelines);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy