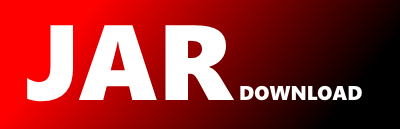
com.metaeffekt.artifact.enrichment.other.timeline.VulnerabilityTimelineGeneratorResult Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.other.timeline;
import com.metaeffekt.artifact.analysis.vulnerability.CommonEnumerationUtil;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.tuple.Pair;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import us.springett.parsers.cpe.Cpe;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
@Slf4j
public class VulnerabilityTimelineGeneratorResult {
private final List timelines;
public VulnerabilityTimelineGeneratorResult(List timelines) {
if (timelines == null) {
log.warn("timelines is null in {}. Creating empty list.", getClass().getSimpleName());
this.timelines = new ArrayList<>();
} else {
this.timelines = timelines.stream().filter(Objects::nonNull).collect(Collectors.toList());
}
}
public VulnerabilityTimelineGeneratorResult() {
this.timelines = new ArrayList<>();
}
public List getTimelinesForArtifact(Artifact artifact, String vulnerabilityId) {
if (vulnerabilityId == null) {
log.warn("vulnerabilityId is null in {}. Returning empty list for artifact {}", getClass().getSimpleName(), artifact != null ? artifact.getId() : null);
return new ArrayList<>();
}
final List cpeUrisOnArtifact = CommonEnumerationUtil.parseEffectiveCpe(artifact);
final List> vendorProductsOnArtifact = CommonEnumerationUtil.getVendorProducts(cpeUrisOnArtifact);
final List result = new ArrayList<>();
for (VulnerabilityTimeline timeline : timelines) {
if (timeline == null) continue;
if (timeline.containsVulnerability(vulnerabilityId)) {
addTimelineToResultWhenMatching(timeline, vendorProductsOnArtifact, result);
}
}
if (result.isEmpty()) {
// return first that matches the vendor product
for (VulnerabilityTimeline timeline : timelines) {
if (timeline == null) continue;
addTimelineToResultWhenMatching(timeline, vendorProductsOnArtifact, result);
}
// limit to 2
if (result.size() > 2) {
result.subList(2, result.size()).clear();
}
}
return result;
}
private void addTimelineToResultWhenMatching(VulnerabilityTimeline timeline,
List> vendorProductsOnArtifact, List result) {
if (timeline == null) return;
final String vendor = timeline.getVendor();
final String product = timeline.getProduct();
if (vendor != null && product != null) {
for (final Pair vp : vendorProductsOnArtifact) {
if (vendor.equals(vp.getLeft()) && product.equals(vp.getRight())) {
result.add(timeline);
break;
}
}
}
}
public List getTimelinesForArtifacts(Collection artifacts, String vulnerabilityId) {
final List result = new ArrayList<>();
for (Artifact artifact : artifacts) {
result.addAll(getTimelinesForArtifact(artifact, vulnerabilityId));
}
return result.stream()
.distinct()
.collect(Collectors.toList());
}
public List getTimelines() {
return timelines;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy