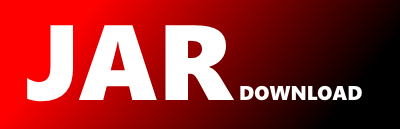
com.metaeffekt.artifact.enrichment.vulnerability.VulnerabilityKeywordsEnrichment Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.enrichment.vulnerability;
import com.metaeffekt.artifact.analysis.vulnerability.enrichment.InventoryAttribute;
import com.metaeffekt.artifact.analysis.vulnerability.enrichment.keywords.KeywordSet;
import com.metaeffekt.artifact.analysis.vulnerability.enrichment.keywords.VulnerabilityKeywords;
import com.metaeffekt.artifact.analysis.vulnerability.enrichment.vulnerabilitystatus.VulnerabilityStatus;
import com.metaeffekt.artifact.enrichment.InventoryEnricher;
import com.metaeffekt.artifact.enrichment.configurations.VulnerabilityKeywordsEnrichmentConfiguration;
import com.metaeffekt.artifact.enrichment.other.vad.VulnerabilityAssessmentDashboard;
import com.metaeffekt.mirror.contents.base.VulnerabilityContextInventory;
import com.metaeffekt.mirror.contents.vulnerability.Vulnerability;
import com.metaeffekt.mirror.download.documentation.EnricherMetadata;
import com.metaeffekt.mirror.download.documentation.InventoryEnrichmentPhase;
import org.json.JSONArray;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.List;
import java.util.stream.Collectors;
@EnricherMetadata(
name = "Vulnerability Keywords", phase = InventoryEnrichmentPhase.ASSESSMENTS,
intermediateFileSuffix = "vulnerability-keywords", mavenPropertyName = "vulnerabilityKeywordsEnrichment"
)
public class VulnerabilityKeywordsEnrichment extends InventoryEnricher {
private static final Logger LOG = LoggerFactory.getLogger(VulnerabilityKeywordsEnrichment.class);
private VulnerabilityKeywordsEnrichmentConfiguration configuration = new VulnerabilityKeywordsEnrichmentConfiguration();
public void setConfiguration(VulnerabilityKeywordsEnrichmentConfiguration configuration) {
this.configuration = configuration;
}
@Override
public VulnerabilityKeywordsEnrichmentConfiguration getConfiguration() {
return configuration;
}
@Override
protected void performEnrichment(Inventory inventory) {
LOG.info("Performing enrichment using [{}] yaml file{}", configuration.getYamlFiles().size(), configuration.getYamlFiles().size() == 1 ? "" : "s");
final VulnerabilityContextInventory vInventory = VulnerabilityContextInventory.fromInventory(inventory);
final VulnerabilityKeywords keywords = configuration.makeKeywords(super.getSecurityPolicyConfiguration().getJsonSchemaValidationErrorsHandling());
for (Vulnerability vulnerability : vInventory.getVulnerabilities()) {
final List matchingKeywords = keywords.getMatching(
vulnerability.getDescription(),
String.join(", ", vulnerability.getCwes())
);
if (!matchingKeywords.isEmpty()) {
LOG.debug("[{}] matches [{}] keyword(s): {}", vulnerability.getId(), matchingKeywords.size(), matchingKeywords.stream().map(KeywordSet::getNameScore).collect(Collectors.joining(", ")));
final boolean anyKeywordSetHasScore = matchingKeywords.stream().anyMatch(KeywordSet::hasScore);
final Double totalKeywordsScore = anyKeywordSetHasScore
? matchingKeywords.stream().filter(KeywordSet::hasScore).mapToDouble(KeywordSet::getScore).sum()
: null;
vulnerability.setAdditionalAttribute(InventoryAttribute.KEYWORDS_SCORE, totalKeywordsScore != null ? String.valueOf(VulnerabilityAssessmentDashboard.roundOneDecimal(totalKeywordsScore)) : null);
final JSONArray keywordsArray = KeywordSet.toFullInformationJson(matchingKeywords);
vulnerability.setAdditionalAttribute(InventoryAttribute.KEYWORDS, keywordsArray.toString());
final VulnerabilityStatus status = findApplicableStatusFromKeywordSets(matchingKeywords);
if (status != null) {
status.appendToVulnerabilityStatus(vulnerability.getOrCreateNewVulnerabilityStatus(), configuration.getActiveLabels());
vulnerability.getVulnerabilityStatus().applyToVulnerability(vulnerability);
}
}
}
vInventory.writeBack(true);
}
private VulnerabilityStatus findApplicableStatusFromKeywordSets(List keywords) {
final List keywordsWithStatus = keywords.stream().filter(e -> e.getStatus() != null).collect(Collectors.toList());
final VulnerabilityStatus status;
if (keywordsWithStatus.size() > 1) {
status = keywordsWithStatus.stream().map(KeywordSet::getStatus).findFirst().orElse(null);
LOG.warn("Matched keywords contain [{}] vulnerability statuses, picking random entry [{}]", keywordsWithStatus.size(), status);
} else if (keywordsWithStatus.size() == 1) {
status = keywordsWithStatus.get(0).getStatus();
} else {
status = null;
}
return status;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy