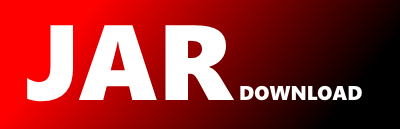
com.metaeffekt.mirror.contents.advisory.GeneralAdvisorEntry Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.contents.advisory;
import com.metaeffekt.mirror.contents.store.AdvisoryTypeIdentifier;
import com.metaeffekt.mirror.contents.store.AdvisoryTypeStore;
import lombok.Setter;
import org.apache.commons.lang3.StringUtils;
import org.apache.lucene.document.Document;
import org.json.JSONObject;
import org.metaeffekt.core.inventory.processor.model.AdvisoryMetaData;
import org.metaeffekt.core.inventory.processor.report.model.AdvisoryUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
public class GeneralAdvisorEntry extends AdvisoryEntry {
private final static Logger LOG = LoggerFactory.getLogger(GeneralAdvisorEntry.class);
protected final static Set CONVERSION_KEYS_AMB = new HashSet(AdvisoryEntry.CONVERSION_KEYS_AMB) {{
}};
protected final static Set CONVERSION_KEYS_MAP = new HashSet(AdvisoryEntry.CONVERSION_KEYS_MAP) {{
}};
@Setter
private String url;
@Setter
private String type = "alert";
public GeneralAdvisorEntry() {
super(AdvisoryTypeStore.get().fromNameAndImplementation("UNKNOWN", "UNKNOWN"));
}
public GeneralAdvisorEntry(String id) {
super(AdvisoryTypeStore.get().fromNameAndImplementation("UNKNOWN", "UNKNOWN"), id);
}
public GeneralAdvisorEntry(AdvisoryTypeIdentifier> source) {
super(source);
}
public GeneralAdvisorEntry(AdvisoryTypeIdentifier> source, String id) {
super(source, id);
}
@Override
public String getUrl() {
return url;
}
@Override
public String getType() {
return AdvisoryUtils.normalizeType(type);
}
/* TYPE CONVERSION METHODS */
@Override
protected Set conversionKeysAmb() {
return CONVERSION_KEYS_AMB;
}
@Override
protected Set conversionKeysMap() {
return CONVERSION_KEYS_MAP;
}
@Override
public GeneralAdvisorEntry constructDataClass() {
return new GeneralAdvisorEntry();
}
public static GeneralAdvisorEntry fromAdvisoryMetaData(AdvisoryMetaData amd) {
return AdvisoryEntry.fromAdvisoryMetaData(amd, GeneralAdvisorEntry::new);
}
public static GeneralAdvisorEntry fromInputMap(Map map) {
return AdvisoryEntry.fromInputMap(map, GeneralAdvisorEntry::new);
}
public static GeneralAdvisorEntry fromJson(JSONObject json) {
return AdvisoryEntry.fromJson(json, GeneralAdvisorEntry::new);
}
public static GeneralAdvisorEntry fromDocument(Document document) {
return AdvisoryEntry.fromDocument(document, GeneralAdvisorEntry::new);
}
protected static String stringOrNull(Object obj) {
if (obj == null) {
return null;
}
return obj.toString();
}
@Override
public void appendFromBaseModel(AdvisoryMetaData amd) {
super.appendFromBaseModel(amd);
this.url = stringOrNull(amd.get(AdvisoryMetaData.Attribute.URL));
if (StringUtils.isNotEmpty(amd.get(AdvisoryMetaData.Attribute.TYPE))) {
this.type = String.valueOf(amd.get(AdvisoryMetaData.Attribute.TYPE));
}
}
@Override
public void appendToBaseModel(AdvisoryMetaData amd) {
super.appendToBaseModel(amd);
}
@Override
public void appendFromMap(Map map) {
super.appendFromMap(map);
this.url = stringOrNull(map.get("url"));
if (StringUtils.isNotEmpty(String.valueOf(map.get("type")))) {
this.type = String.valueOf(map.get("type"));
}
}
@Override
public void appendToJson(JSONObject json) {
super.appendToJson(json);
}
@Override
public void appendFromDocument(Document document) {
super.appendFromDocument(document);
}
@Override
public void appendToDocument(Document document) {
super.appendToDocument(document);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy