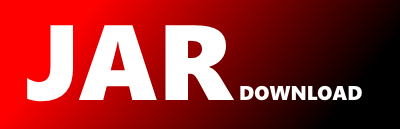
com.metaeffekt.mirror.contents.base.AmbDataClass Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.contents.base;
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field.Store;
import org.apache.lucene.document.TextField;
import org.json.JSONObject;
import org.metaeffekt.core.inventory.processor.model.AbstractModelBase;
import org.metaeffekt.core.inventory.processor.model.AdvisoryMetaData;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.VulnerabilityMetaData;
import java.util.*;
import java.util.function.Consumer;
public abstract class AmbDataClass> implements Comparable> {
protected final static Set CONVERSION_KEYS_AMB = new HashSet<>(Arrays.asList(
Artifact.Attribute.ID.getKey(),
VulnerabilityMetaData.Attribute.NAME.getKey(),
AdvisoryMetaData.Attribute.NAME.getKey()
));
protected final static Set CONVERSION_KEYS_MAP = new HashSet<>(Arrays.asList(
"id"
));
protected String id;
protected Map additionalAttributes = new LinkedHashMap<>();
public Map getAdditionalAttributes() {
return additionalAttributes;
}
public void setAdditionalAttribute(String key, String value) {
if (value == null) {
additionalAttributes.remove(key);
} else {
additionalAttributes.put(key, value);
}
}
public void setAdditionalAttribute(T key, String value) {
if (value == null) {
additionalAttributes.remove(key.getKey());
} else {
additionalAttributes.put(key.getKey(), value);
}
}
public String getAdditionalAttribute(String key) {
return additionalAttributes.get(key);
}
public String getAdditionalAttribute(T key) {
return additionalAttributes.get(key.getKey());
}
public String getId() {
return id;
}
public DC setId(String id) {
this.id = id;
return (DC) this;
}
public abstract AMB constructBaseModel();
public abstract DC constructDataClass();
protected abstract Set conversionKeysAmb();
protected abstract Set conversionKeysMap();
public void appendFromDataClass(DC dataClass) {
this.additionalAttributes.putAll(dataClass.getAdditionalAttributes());
this.setId(dataClass.getId());
}
protected void appendToBaseModel(AMB baseModel) {
for (Map.Entry attributes : additionalAttributes.entrySet()) {
baseModel.set(attributes.getKey(), attributes.getValue());
}
if (baseModel instanceof VulnerabilityMetaData) {
baseModel.set(VulnerabilityMetaData.Attribute.NAME.getKey(), this.getId());
} else if (baseModel instanceof AdvisoryMetaData) {
baseModel.set(AdvisoryMetaData.Attribute.NAME.getKey(), this.getId());
} else if (baseModel instanceof Artifact) {
baseModel.set(Artifact.Attribute.ID.getKey(), this.getId());
} else {
baseModel.set("Id", this.getId());
}
}
/**
* Appends the data from a base model instance to this instance, overwriting existing values.
* Returns a set of keys that have been used to overwrite values.
*
* @param baseModel The base model instance to append from.
*/
protected void appendFromBaseModel(AMB baseModel) {
final Set modelKeys = conversionKeysAmb();
baseModel.getAttributes().stream()
.filter(a -> !modelKeys.contains(a))
.forEach(a -> this.getAdditionalAttributes().put(a, baseModel.get(a)));
if (baseModel instanceof VulnerabilityMetaData) {
this.setId(baseModel.get(VulnerabilityMetaData.Attribute.NAME));
} else if (baseModel instanceof AdvisoryMetaData) {
this.setId(baseModel.get(AdvisoryMetaData.Attribute.NAME));
} else if (baseModel instanceof Artifact) {
this.setId(baseModel.get(Artifact.Attribute.ID));
} else {
this.setId(baseModel.get("Id"));
}
}
protected void appendToJson(JSONObject json) {
for (Map.Entry attributes : additionalAttributes.entrySet()) {
json.put(attributes.getKey(), attributes.getValue());
}
json.put("id", this.getId());
}
protected void appendFromMap(Map map) {
final Set modelKeys = conversionKeysMap();
map.keySet().stream()
.filter(a -> !modelKeys.contains(a))
.forEach(a -> this.getAdditionalAttributes().put(a, String.valueOf(map.get(a))));
if (map.containsKey("id")) {
this.setId(String.valueOf(map.get("id")));
}
}
protected void appendToDocument(Document document) {
if (this.getId() == null) {
throw new IllegalStateException("The ID of an advisory entry must not be null when converting to a lucene document.");
}
document.add(new TextField("id", this.getId(), Store.YES));
}
protected void appendFromDocument(Document document) {
this.setId(document.get("id"));
}
public AMB toBaseModel() {
final AMB amb = constructBaseModel();
appendToBaseModel(amb);
return amb;
}
public JSONObject toJson() {
final JSONObject json = new JSONObject();
appendToJson(json);
return json;
}
public Document toDocument() {
final Document document = new Document();
appendToDocument(document);
return document;
}
public T performAction(Consumer action) {
action.accept((T) this);
return (T) this;
}
@Override
public int compareTo(AmbDataClass o) {
if (this.getId() == null && o.getId() == null) return 0;
if (this.getId() == null) return -1;
if (o.getId() == null) return 1;
return String.CASE_INSENSITIVE_ORDER.compare(this.getId(), o.getId());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy