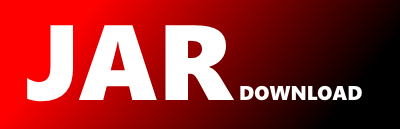
com.metaeffekt.mirror.contents.base.DescriptionParagraph Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.contents.base;
import com.metaeffekt.artifact.analysis.utils.StringUtils;
import j2html.tags.specialized.PTag;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.List;
import static j2html.TagCreator.*;
public class DescriptionParagraph {
private final String header;
private final String content;
public DescriptionParagraph(String header, String content) {
this.header = StringUtils.isEmpty(header) ? null : header;
this.content = StringUtils.isEmpty(content) ? null : content;
}
public String getHeader() {
return header;
}
public String getContent() {
return content;
}
public boolean isEmpty() {
return this.header == null && this.content == null;
}
@Override
public String toString() {
return toMarkdownString(1);
}
public String toMarkdownString(int header) {
final StringBuilder headerBuilder = new StringBuilder();
final boolean headerExists = StringUtils.hasText(this.header);
final boolean contentExists = StringUtils.hasText(this.content);
if (headerExists) {
for (int i = 0; i < header; i++) {
headerBuilder.append("#");
}
}
headerBuilder
.append(headerExists ? " " : "")
.append(headerExists ? this.header : "")
.append(headerExists && contentExists ? "\n" : "")
.append(contentExists ? this.content : "");
return headerBuilder.toString();
}
public PTag toHtmlH1Tag() {
return p()
.with(h1(this.header))
.with(text(this.content));
}
public JSONObject toJson() {
return new JSONObject()
.put("header", this.header)
.put("content", this.content);
}
public static DescriptionParagraph fromTitleAndContent(String title, String content) {
return new DescriptionParagraph(title, content);
}
public static DescriptionParagraph fromTitle(String title) {
return new DescriptionParagraph(title, null);
}
public static DescriptionParagraph fromContent(String content) {
return new DescriptionParagraph(null, content);
}
public static DescriptionParagraph fromJson(JSONObject json) {
return new DescriptionParagraph(
json.optString("header", null),
json.optString("content", null)
);
}
public static List fromJson(JSONArray json) {
final List paragraphs = new ArrayList<>();
if (json == null) return paragraphs;
for (int i = 0; i < json.length(); i++) {
paragraphs.add(fromJson(json.optJSONObject(i)));
}
return paragraphs;
}
public DescriptionParagraph deriveWithHeader(String header) {
return new DescriptionParagraph(header, this.content);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy