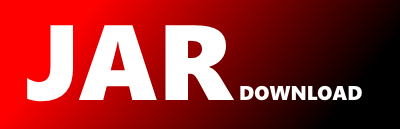
com.metaeffekt.mirror.contents.base.Reference Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.contents.base;
import com.metaeffekt.artifact.analysis.utils.StringUtils;
import j2html.TagCreator;
import j2html.tags.specialized.ATag;
import org.apache.commons.lang3.ObjectUtils;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.*;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
public class Reference {
public final static Pattern REFERENCE_STRING_PATTERN = Pattern.compile("^([^:]+): ([^(]+) \\((.+)\\)$");
private final String title;
private final String url;
private final Set tags = new LinkedHashSet<>();
public Reference(String title, String url) {
if (StringUtils.isEmpty(title) || title.equals("null")) this.title = null;
else this.title = title;
if (StringUtils.isEmpty(url) || url.equals("null")) this.url = null;
else this.url = url;
}
public void addTag(String tag) {
tags.add(tag);
}
public void removeTag(String tag) {
tags.remove(tag);
}
public String getTitle() {
return title;
}
public String getUrl() {
return url;
}
public Set getTags() {
return tags;
}
@Override
public String toString() {
return toMarkdownString();
}
public JSONObject toJson() {
final JSONObject json = new JSONObject();
json.put("title", title);
json.put("url", url);
if (!tags.isEmpty()) {
json.put("tags", tags);
}
return json;
}
private String getTaggedTitle() {
return (title != null ? title : url) + (!tags.isEmpty() ? " (" + String.join(", ", tags) + ")" : "");
}
public String toMarkdownString() {
return "[" + getTaggedTitle() + "](" + url + ")";
}
public String toDisplayString() {
if (getTaggedTitle().equals("null")) {
return url;
}
return getTaggedTitle() + " " + url;
}
public ATag toHtmlATag() {
return TagCreator.a()
.withHref(url == null ? "#" : url)
.withText(title == null ? url : getTaggedTitle());
}
public static Reference fromJson(JSONObject json) {
if (json == null) return null;
final Reference ref = new Reference(
ObjectUtils.firstNonNull(json.optString("title", null), json.optString("type", null)),
ObjectUtils.firstNonNull(json.optString("url", null), json.optString("ref", null))
);
final JSONArray tags = json.optJSONArray("tags");
if (tags != null) tags.forEach(tag -> ref.addTag(tag.toString()));
return ref;
}
public static Reference fromMap(Map map) {
final String name = String.valueOf(map.getOrDefault("title", null));
final String url = String.valueOf(map.getOrDefault("url", null));
final Reference ref = new Reference(name, url);
final Object tags = map.getOrDefault("tags", null);
if (tags instanceof Collection) {
for (String tag : ((Collection) tags)) {
ref.addTag(tag);
}
}
return ref;
}
public static Reference fromUrl(String url) {
return new Reference(
null,
url
);
}
public static Reference fromUrlAndTags(String url, String... tags) {
final Reference reference = new Reference(
null,
url
);
for (String tag : tags) {
reference.addTag(tag);
}
return reference;
}
public static Reference fromTitle(String title) {
return new Reference(
title,
null
);
}
public static Reference fromTitleAndUrl(String title, String url) {
return new Reference(
title,
url
);
}
public static List fromJsonArray(JSONArray json) {
if (json == null) return new ArrayList<>();
return IntStream.range(0, json.length())
.mapToObj(i -> {
final JSONObject entry = json.optJSONObject(i);
if (entry == null) {
return handleStringCase(json.optString(i, null));
}
return fromJson(entry);
})
.filter(Objects::nonNull)
.collect(Collectors.toList());
}
private static Reference handleStringCase(String str) {
if (str != null) {
final String[] parts = str.split(" \\(");
if (parts.length == 2) {
return new Reference(parts[0], parts[1].substring(0, parts[1].length() - 1));
} else {
if (str.startsWith("http")) {
return new Reference(null, str);
} else {
return new Reference(str, null);
}
}
}
return null;
}
public static List fromJsonArray(String jsonString) {
if (jsonString == null || !jsonString.startsWith("[")) return new ArrayList<>();
return fromJsonArray(new JSONArray(jsonString));
}
public static List mergeReferences(Collection... references) {
final List merged = new ArrayList<>();
for (Collection referenceSet : references) {
for (Reference reference : referenceSet) {
final Reference existing = merged.stream()
.filter(r -> r.getUrl() != null && r.getUrl().equals(reference.getUrl()))
.findFirst()
.orElse(null);
if (existing != null) {
existing.getTags().addAll(reference.getTags());
} else {
merged.add(reference);
}
}
}
return merged;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy