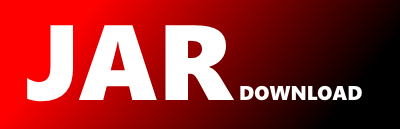
com.metaeffekt.mirror.contents.cpe.NvdCpeMetadata Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.contents.cpe;
import com.metaeffekt.artifact.analysis.vulnerability.CommonEnumerationUtil;
import com.metaeffekt.mirror.contents.base.Reference;
import org.apache.lucene.document.Document;
import org.json.JSONArray;
import org.json.JSONObject;
import us.springett.parsers.cpe.Cpe;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
public class NvdCpeMetadata {
private final static String NVD_BASE_URL = "https://nvd.nist.gov/products/cpe/detail/";
private final Cpe cpe;
private final boolean deprecated;
private final String nvdId;
private final Map titles;
private final List references;
public NvdCpeMetadata(Cpe cpe, boolean deprecated, String nvdId, Map titles, List references) {
this.cpe = cpe;
this.deprecated = deprecated;
this.nvdId = nvdId;
this.titles = titles;
this.references = references;
}
public NvdCpeMetadata(Document document) {
if (document == null) {
throw new IllegalArgumentException("Document must not be null.");
}
if (document.getField("cpe23Uri") != null) {
this.cpe = CommonEnumerationUtil.parseCpe(document.getField("cpe23Uri").stringValue()).orElse(null);
} else {
this.cpe = null;
}
this.deprecated = document.getField("deprecated") != null && document.getField("deprecated").stringValue().equals("true");
this.nvdId = document.getField("nvdId") != null ? document.getField("nvdId").stringValue() : null;
this.titles = document.getField("titles") != null
? parseTitles(document.getField("titles").stringValue())
: new HashMap<>();
this.references = document.getField("references") != null
? Reference.fromJsonArray(new JSONArray(document.getField("references").stringValue()))
: new ArrayList<>();
}
private Map parseTitles(String titles) {
if (titles.startsWith("[")) {
final JSONArray titlesArray = new JSONArray(titles);
return IntStream.range(0, titlesArray.length())
.mapToObj(titlesArray::getJSONObject)
.collect(Collectors.toMap(
title -> title.getString("lang"),
title -> title.getString("title"),
(a, b) -> a
));
} else {
return new HashMap<>();
}
}
public Cpe getCpe() {
return cpe;
}
public boolean isDeprecated() {
return deprecated;
}
public String getNvdId() {
return nvdId;
}
public Map getTitles() {
return titles;
}
public String getEnglishTitleOrFallbackAny() {
if (titles.containsKey("en")) {
return titles.get("en");
} else if (!titles.isEmpty()) {
return titles.values().iterator().next();
} else {
return null;
}
}
public List getReferences() {
return references;
}
public String toUrl() {
return NVD_BASE_URL + nvdId;
}
public JSONObject toJson() {
return new JSONObject()
.put("cpe", cpe != null ? CommonEnumerationUtil.toCpe22UriOrFallbackToCpe23FS(cpe) : null)
.put("deprecated", deprecated)
.put("nvdId", nvdId)
.put("titles", titles)
.put("references", references.stream().map(Reference::toJson).collect(Collectors.toList()));
}
@Override
public String toString() {
return "NvdCpeMetadata{" +
"cpe=" + cpe +
", deprecated=" + deprecated +
", nvdId='" + nvdId + '\'' +
", titles=" + titles +
", references=" + references +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy