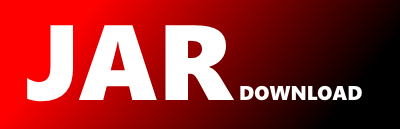
com.metaeffekt.mirror.contents.epss.EpssData Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.contents.epss;
import lombok.*;
import lombok.extern.slf4j.Slf4j;
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field;
import org.apache.lucene.document.TextField;
import org.json.JSONObject;
import java.util.Locale;
@Slf4j
@Getter
@AllArgsConstructor
@Setter(AccessLevel.PRIVATE)
@NoArgsConstructor(access = AccessLevel.PUBLIC)
@EqualsAndHashCode
public class EpssData {
private String vulnerability;
private float epssScore;
private float percentile;
public EpssData(String vulnerability, double epssScore, double percentile) {
this.vulnerability = vulnerability;
this.epssScore = (float) epssScore;
this.percentile = (float) percentile;
}
public static EpssData fromJson(JSONObject json) {
if (json == null) {
return null;
}
final EpssData epssData = new EpssData();
epssData.setVulnerability((String) json.get("vulnerability"));
epssData.setEpssScore(parseFloat(json.get("epssScore")));
epssData.setPercentile(parseFloat(json.get("percentile")));
return epssData;
}
public JSONObject toJson() {
final JSONObject json = new JSONObject();
json.put("vulnerability", vulnerability);
json.put("epssScore", epssScore);
json.put("percentile", percentile);
return json;
}
public static EpssData fromDocument(Document document) {
final EpssData epssData = new EpssData();
epssData.setVulnerability(document.get("vulnerability"));
epssData.setEpssScore(parseFloat(document.get("epssScore")));
epssData.setPercentile(parseFloat(document.get("percentile")));
return epssData;
}
public Document toDocument() {
final Document doc = new Document();
doc.add(new TextField("vulnerability", vulnerability, Field.Store.YES));
doc.add(new TextField("epssScore", String.format(Locale.ENGLISH, "%f", epssScore), Field.Store.YES));
doc.add(new TextField("percentile", String.format(Locale.ENGLISH, "%f", percentile), Field.Store.YES));
return doc;
}
private static float parseFloat(Object value) {
if (value == null) {
return 0;
} else if (value instanceof Number) {
return ((Number) value).floatValue();
} else if (!(value instanceof String)) {
return 0;
}
final String stringValue = (String) value;
// check for both "," and "." as decimal separator
try {
return Float.parseFloat(stringValue);
} catch (NumberFormatException e) {
return Float.parseFloat(stringValue.replace(",", "."));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy