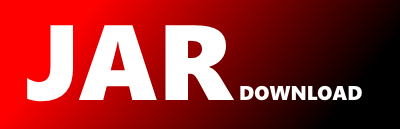
com.metaeffekt.mirror.contents.msrcdata.MsrcRemediation Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.contents.msrcdata;
import com.metaeffekt.mirror.contents.base.Reference;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.*;
import java.util.stream.Collectors;
public class MsrcRemediation implements Comparable {
private final String type;
private final String subType;
private final String description;
private final Reference url;
private final Set affectedProductIds;
private final String fixedBuild;
private final String supercedence;
public MsrcRemediation(String type, String subType, String description, Reference url, Set affectedProductIds, String fixedBuild, String supercedence) {
this.type = type;
this.subType = subType;
this.description = description;
this.url = url;
this.affectedProductIds = affectedProductIds;
this.fixedBuild = fixedBuild;
this.supercedence = supercedence;
}
public String getType() {
return type;
}
public String getSubType() {
return subType;
}
public String getDescription() {
return description;
}
public Reference getUrl() {
return url;
}
public Set getAffectedProductIds() {
return affectedProductIds;
}
public String getFixedBuild() {
return fixedBuild;
}
public String getSupercedence() {
return supercedence;
}
public JSONObject toJson() {
final JSONObject json = new JSONObject();
json.put("type", type);
json.put("subType", subType);
json.put("description", description);
json.put("url", url == null ? null : url.toJson());
json.put("affectedProductIds", new JSONArray(affectedProductIds));
json.put("fixedBuild", fixedBuild);
json.put("supercedence", supercedence);
return json;
}
public static MsrcRemediation fromJson(JSONObject json) {
return new MsrcRemediation(
json.optString("type", null),
json.optString("subType", null),
json.optString("description", null),
json.has("url") ? Reference.fromJson(json.getJSONObject("url")) : null,
json.getJSONArray("affectedProductIds").toList().stream().map(Object::toString).collect(Collectors.toSet()),
json.optString("fixedBuild", null),
json.optString("supercedence", null)
);
}
public static MsrcRemediation fromMap(Map map) {
return new MsrcRemediation(
(String) map.get("type"),
(String) map.get("subType"),
(String) map.get("description"),
map.containsKey("url") ? Reference.fromMap((Map) map.get("url")) : null,
((List) map.get("affectedProductIds")).stream().collect(Collectors.toSet()),
(String) map.get("fixedBuild"),
(String) map.get("supercedence")
);
}
public static List fromJson(JSONArray json) {
final List threats = new ArrayList<>();
for (Object object : json) {
threats.add(fromJson((JSONObject) object));
}
return threats;
}
public static List fromMap(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy