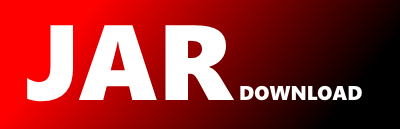
com.metaeffekt.mirror.contents.vulnerability.VulnerableSoftwareVersionRange Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.contents.vulnerability;
import com.metaeffekt.artifact.analysis.utils.StringUtils;
import com.metaeffekt.artifact.analysis.version.Version;
import com.metaeffekt.artifact.analysis.version.curation.VersionContext;
import org.json.JSONObject;
public class VulnerableSoftwareVersionRange {
protected final String version;
protected final String update;
protected final String versionEndExcluding;
protected final String versionEndIncluding;
protected final String versionStartExcluding;
protected final String versionStartIncluding;
protected final boolean vulnerable;
protected final VersionContext context;
public VulnerableSoftwareVersionRange(String version, String update, String versionStartExcluding, String versionStartIncluding, String versionEndIncluding, String versionEndExcluding, VersionContext context, boolean vulnerable) {
this.version = version;
this.update = update;
this.versionEndExcluding = versionEndExcluding;
this.versionEndIncluding = versionEndIncluding;
this.versionStartExcluding = versionStartExcluding;
this.versionStartIncluding = versionStartIncluding;
this.context = context == null ? VersionContext.EMPTY : context;
this.vulnerable = vulnerable;
}
public String getVersionEndExcluding() {
return versionEndExcluding;
}
public String getVersionEndIncluding() {
return versionEndIncluding;
}
public String getVersionStartExcluding() {
return versionStartExcluding;
}
public String getVersionStartIncluding() {
return versionStartIncluding;
}
public String getVersion() {
return version;
}
public String getUpdate() {
return update;
}
public VersionContext getContext() {
return context;
}
public boolean isVulnerable() {
return vulnerable;
}
protected boolean isVersionRangeSpecified() {
return versionEndExcluding != null || versionEndIncluding != null || versionStartExcluding != null || versionStartIncluding != null;
}
public boolean matches(Version version) {
return version.matchesVersionOf(this);
}
public JSONObject toJson() {
return new JSONObject()
.put("version", version)
.put("update", update)
.put("versionEndExcluding", versionEndExcluding)
.put("versionEndIncluding", versionEndIncluding)
.put("versionStartExcluding", versionStartExcluding)
.put("versionStartIncluding", versionStartIncluding)
.put("vulnerable", vulnerable)
.put("context", context.toJson());
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
final boolean versionSpecified = StringUtils.hasText(version);
final boolean updateSpecified = StringUtils.hasText(update);
final boolean versionAny = versionSpecified && version.equals("*");
final boolean updateAny = updateSpecified && update.equals("*");
final boolean isNotBothAny = !(versionAny && updateAny);
if (isNotBothAny) {
if (versionSpecified) {
sb.append(version);
}
if (updateSpecified) {
sb.append(" (").append(update).append(")");
}
}
if (isVersionRangeSpecified()) {
if (versionStartExcluding != null) {
sb.append("(").append(versionStartExcluding).append(", ");
} else if (versionStartIncluding != null) {
sb.append("[").append(versionStartIncluding).append(", ");
} else {
sb.append("[, ");
}
if (versionEndExcluding != null) {
sb.append(versionEndExcluding).append(")");
} else if (versionEndIncluding != null) {
sb.append(versionEndIncluding).append("]");
} else {
sb.append(", ]");
}
}
if (!vulnerable) {
if (sb.length() > 0) {
sb.append(" ");
}
sb.append("(not vulnerable)");
}
return sb.toString();
}
/*public static VulnerableSoftwareVersionRange fromJson(JSONObject json) {
return new VulnerableSoftwareVersionRange(
json.optString("version", null),
json.optString("update", null),
json.optString("versionStartExcluding", null), json.optString("versionStartIncluding", null), json.optString("versionEndIncluding", null), json.optString("versionEndExcluding", null),
VersionContext.EMPTY,
json.optBoolean("vulnerable", true)
);
}*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy