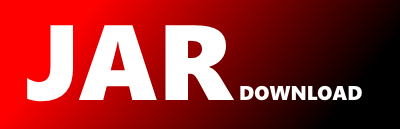
com.metaeffekt.mirror.download.GitRepositoryDownload Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.download;
import com.metaeffekt.artifact.analysis.utils.ArchiveUtils;
import com.metaeffekt.artifact.analysis.utils.FileUtils;
import com.metaeffekt.mirror.download.documentation.DocRelevantMethods;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.util.ArrayList;
public abstract class GitRepositoryDownload extends Download {
private final static Logger LOG = LoggerFactory.getLogger(GitRepositoryDownload.class);
private boolean useGitCommand = false;
public GitRepositoryDownload(File baseMirrorDirectory, Class extends Download> downloadClass) {
super(baseMirrorDirectory, downloadClass);
}
public GitRepositoryDownload setUseGitCommand(boolean useGitCommand) {
this.useGitCommand = useGitCommand;
return this;
}
@Override
@DocRelevantMethods({"GitRepositoryDownload#performGitDownload", "GitRepositoryDownload#performZipDownload"})
protected void performDownload() {
if (!getDownloadIntoDirectory().exists()) {
getDownloadIntoDirectory().mkdirs();
}
final File dir = new File(getDownloadIntoDirectory(), getRepositoryName());
if (useGitCommand) {
LOG.info("Using git command to clone repository");
performGitDownload(dir);
} else {
LOG.info("Using zip download to download repository");
performZipDownload(dir);
}
LOG.info("Repository updated");
}
private void performZipDownload(File dir) {
final File zipFile = new File(getDownloadIntoDirectory(), getRepositoryName() + ".zip");
LOG.info("[long operation] Downloading [{}] into [{}]", getZipDownloadUrl(), zipFile.getAbsolutePath());
super.downloader.fetchResponseBodyFromUrlToFile(getZipDownloadUrl(), zipFile);
if (dir.exists()) {
LOG.info("[long operation] Deleting existing directory: {}", dir.getAbsolutePath());
try {
FileUtils.deleteDirectory(dir);
} catch (IOException e) {
try {
Thread.sleep(1000);
FileUtils.deleteDirectory(dir);
} catch (IOException | InterruptedException ex) {
throw new RuntimeException("Failed to delete previous download in " + dir.getAbsolutePath(), e);
}
}
}
if (zipFile.exists()) {
LOG.info("[long operation] Unpacking [{}] into [{}]", zipFile.getAbsolutePath(), dir.getParentFile().getAbsolutePath());
ArchiveUtils.unpackIfPossible(zipFile, dir.getParentFile(), new ArrayList<>());
} else {
throw new RuntimeException("Failed to download " + getZipDownloadUrl() + " into " + zipFile.getAbsolutePath());
}
// find the single directory that starts with the target download name and rename it to the target directory
final File[] files = dir.getParentFile().listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory() && file.getName().startsWith(getRepositoryName())) {
LOG.info("Renaming [{}] to [{}]", file.getAbsolutePath(), dir.getAbsolutePath());
file.renameTo(dir);
break;
}
}
}
if (!dir.exists()) {
throw new RuntimeException("Failed to unpack " + zipFile.getAbsolutePath() + " into " + dir.getAbsolutePath());
}
LOG.info("Deleting [{}]", zipFile.getAbsolutePath());
zipFile.delete();
}
private void performGitDownload(File dir) {
final boolean gitLogExists = new File(dir, ".git").exists();
if (gitLogExists) {
try {
LOG.info("Updating repository from {} into {}", getRepositoryUrl(), dir.getAbsolutePath());
super.git.pull(dir);
} catch (IOException e) {
throw new RuntimeException("Failed to pull repository updates from " + getRepositoryUrl() + " into " + dir.getAbsolutePath(), e);
}
} else {
try {
LOG.info("Cloning repository from {} into {}", getRepositoryUrl(), dir.getAbsolutePath());
super.git.cloneRemote(dir, getRepositoryUrl(), null);
} catch (IOException e) {
throw new RuntimeException("Failed to clone repository from " + getRepositoryUrl() + " into " + dir.getAbsolutePath(), e);
}
}
}
@Override
protected boolean additionalIsDownloadRequired() {
return useGitCommand;
}
public abstract String getRepositoryUrl();
public abstract URL getZipDownloadUrl();
private String getRepositoryName() {
return getRepositoryUrl().substring(getRepositoryUrl().lastIndexOf("/") + 1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy