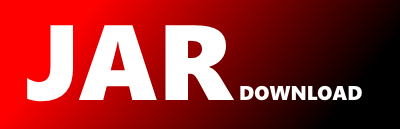
com.metaeffekt.mirror.download.advisor.GhsaDownload Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.download.advisor;
import com.metaeffekt.mirror.download.documentation.MirrorMetadata;
import com.metaeffekt.mirror.download.GitRepositoryDownload;
import com.metaeffekt.mirror.download.ResourceLocation;
import java.io.File;
import java.net.URL;
/**
* References:
*
* - GitHub Security Advisory Database: https://github.com/advisories
*
* GitHub provides a service for project maintainers to publish their own security advisories for their projects. This service
* uses the OSV (Open Source Vulnerabilities) schema to provide structured data on vulnerabilities
* in open source projects. The data is provided in a git repository, which can be cloned or downloaded as a zip file, depending on the useGitCommand
parameter.
* The downloader will create a directory github-advisory-database
in the download
directory, where a directory
* structure will be created that mirrors the structure of the repository.
* The advisories are further split into two directories: advisories/github-reviewed
and advisories/unreviewed
.
* Each file is contained within a separate directory, with the name of the directory being the advisory ID.
* A flag can later be used to indicate to only use reviewed advisories or to also include the unreviewed ones.
* Their schema being the OSV schema, a generalized approach could be useful to mirror potentially many different OSV data sources into the same data structure.
* .
* └── advisories
* ├── github-reviewed
* │ ├── 2017
* │ │ ├── 10
* │ │ │ ├── GHSA-229r-pqp6-8w6g
* │ │ │ │ └── GHSA-229r-pqp6-8w6g.json
* │ │ │ ├── GHSA-24fg-p96v-hxh8
* │ │ │ │ └── GHSA-24fg-p96v-hxh8.json
* └── unreviewed
* ├── 2021
* │ ├── 04
* │ │ └── GHSA-m5pg-8h68-j225
* │ │ └── GHSA-m5pg-8h68-j225.json
*
*/
@MirrorMetadata(directoryName = "github-advisory-database", mavenPropertyName = "githubAdvisorDownload")
public class GhsaDownload extends GitRepositoryDownload {
public GhsaDownload(File baseMirrorDirectory) {
super(baseMirrorDirectory, GhsaDownload.class);
super.maxAgeBeforeReset = 3 * 4L * 7 * 24 * 60 * 60 * 1000; // 3 months
}
@Override
public String getRepositoryUrl() {
return getRemoteResourceLocation(ResourceLocationGHSA.GHSA_GIT_URL);
}
@Override
public URL getZipDownloadUrl() {
return getRemoteResourceLocationUrl(ResourceLocationGHSA.GHSA_GIT_ZIP_DOWNLOAD_URL);
}
@Override
public void setRemoteResourceLocation(String location, String url) {
super.setRemoteResourceLocation(ResourceLocationGHSA.valueOf(location), url);
}
public enum ResourceLocationGHSA implements ResourceLocation {
/**
* Remote repository URL of the
* github/advisory-database repository.
*/
GHSA_GIT_URL("https://github.com/github/advisory-database"),
/**
* Remote URL of the zip download of the
* github/advisory-database repository.
*/
GHSA_GIT_ZIP_DOWNLOAD_URL("https://github.com/github/advisory-database/archive/refs/heads/main.zip");
private final String defaultValue;
ResourceLocationGHSA(String defaultValue) {
this.defaultValue = defaultValue;
}
@Override
public String getDefault() {
return this.defaultValue;
}
}
}