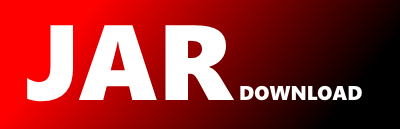
com.metaeffekt.mirror.index.other.EolIndex Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.index.other;
import com.metaeffekt.artifact.analysis.utils.FileUtils;
import com.metaeffekt.mirror.download.documentation.MirrorMetadata;
import com.metaeffekt.mirror.contents.eol.EolLifecycle;
import com.metaeffekt.mirror.download.documentation.DocRelevantMethods;
import com.metaeffekt.mirror.download.other.EolDownload;
import com.metaeffekt.mirror.index.Index;
import org.apache.lucene.document.Document;
import org.json.JSONArray;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
@MirrorMetadata(directoryName = "eol", mavenPropertyName = "eolIndex")
public class EolIndex extends Index {
private final static Logger LOG = LoggerFactory.getLogger(EolIndex.class);
public EolIndex(File baseMirrorDirectory) {
super(baseMirrorDirectory, EolIndex.class, Collections.singletonList(EolDownload.class), Collections.emptyList());
}
@Override
@DocRelevantMethods({"EolLifecycle#fromJson", "EolCycle#fromJson"})
protected Map createIndexDocuments() {
final Map documents = new ConcurrentHashMap<>();
final Collection files = super.getAllFilesRecursively(super.requiredDownloads[0]);
LOG.info("Found [{}] files in repository", files.size());
for (File file : files) {
if (!file.getName().endsWith(".json")) {
continue;
}
final String product = file.getName().replace(".json", "");
try {
final String contents = FileUtils.readFileToString(file, StandardCharsets.UTF_8);
final JSONArray parsedContents = new JSONArray(contents);
final EolLifecycle productInfo = EolLifecycle.fromJson(product, parsedContents);
for (Document document : productInfo.toDocuments()) {
String uldid = product + "-" + document.get("cycle");
if (documents.containsKey(uldid)) {
uldid = uldid + "-" + document.get("link");
if (documents.containsKey(uldid)) {
LOG.warn("Duplicate entry found, skipping: {}", document);
}
} else {
documents.put(uldid, document);
}
}
} catch (IOException e) {
throw new RuntimeException("Failed to read file: " + file.getAbsolutePath(), e);
}
}
if (!files.isEmpty()) {
LOG.info("Average of [{}] cycles per product", documents.size() / files.size());
}
return documents;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy