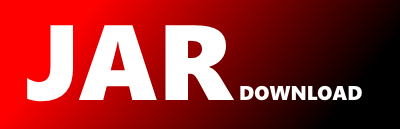
com.metaeffekt.mirror.query.AdvisorIndexQuery Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.query;
import com.metaeffekt.mirror.contents.advisory.AdvisoryEntry;
import com.metaeffekt.mirror.index.Index;
import com.metaeffekt.mirror.index.IndexSearch;
import org.apache.lucene.document.Document;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
public abstract class AdvisorIndexQuery extends IndexQuery {
private List cachedAllAdvisories;
public AdvisorIndexQuery(File baseMirrorDirectory, Class extends Index> index) {
super(baseMirrorDirectory, index);
}
public AdvisorIndexQuery(Index index) {
super(index);
}
public T findById(String id) {
return super.index.findDocuments(new IndexSearch().fieldEquals("id", id)).stream()
.findFirst()
.map(this::createAdvisoryEntry)
.orElse(null);
}
public List findByReferencedId(String referencedId) {
return super.index.findDocuments(new IndexSearch().fieldContains("referencedIds", referencedId)).stream()
.map(this::createAdvisoryEntry)
.filter(entry -> entry.getReferencedVulnerabilities().values().stream().anyMatch(e -> e.contains(referencedId))
|| entry.getReferencedSecurityAdvisories().values().stream().anyMatch(e -> e.contains(referencedId))
|| entry.getReferencedOtherIds().values().stream().anyMatch(e -> e.contains(referencedId)))
.collect(Collectors.toList());
}
public List findByAnyTextFieldContains(String value) {
return super.index.findDocuments(
new IndexSearch()
.fieldContains("summary", value)
.fieldContains("description", value)
.fieldContains("threat", value)
.fieldContains("recommendations", value)
.fieldContains("workarounds", value)
.fieldContains("references", value)
.fieldContains("keywords", value)
.joinOperator("OR")
).stream()
.map(this::createAdvisoryEntry)
.collect(Collectors.toList());
}
public List findCreatedOrUpdatedInRange(long start, long end) {
return findAll().stream()
.filter(entry -> entry.hasBeenUpdatedSince(start) && entry.hasBeenUpdatedBefore(end))
.collect(Collectors.toList());
}
public List findAll() {
if (cachedAllAdvisories == null) {
cachedAllAdvisories = super.index.findAllDocuments().stream()
.map(this::createAdvisoryEntry)
.collect(Collectors.toList());
}
return new ArrayList<>(cachedAllAdvisories);
}
public void findAndProcessAll(Consumer consumer) {
super.index.findAndProcessAllDocuments(document -> consumer.accept(createAdvisoryEntry(document)));
}
public void findAndProcessAllCancelable(Function consumer) {
super.index.findAndProcessAllDocumentsCancelable(document -> consumer.apply(createAdvisoryEntry(document)));
}
protected abstract T createAdvisoryEntry(Document document);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy