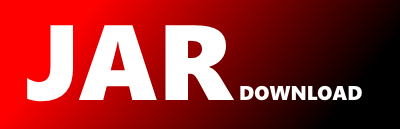
com.metaeffekt.mirror.query.CpeDictionaryVendorProductIndexQuery Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.query;
import com.metaeffekt.mirror.index.IndexSearch;
import com.metaeffekt.mirror.index.nvd.CpeDictionaryVendorProductIndex;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.*;
import java.util.stream.Collectors;
@Deprecated
public class CpeDictionaryVendorProductIndexQuery extends IndexQuery {
private final static Logger LOG = LoggerFactory.getLogger(CpeDictionaryVendorProductIndexQuery.class);
/**
* Maps vendor names to product names.
*/
private final Map> vendorProductsMap = new HashMap<>();
/**
* Maps product names to vendor names.
*/
private final Map> productVendorsMap = new HashMap<>();
public CpeDictionaryVendorProductIndexQuery(File baseMirrorDirectory) {
super(baseMirrorDirectory, CpeDictionaryVendorProductIndex.class);
}
public CpeDictionaryVendorProductIndexQuery(CpeDictionaryVendorProductIndex index) {
super(index);
}
public boolean vendorExists(String vendor) {
return super.index.findDocuments(new IndexSearch().fieldEquals("vendor", vendor).fieldEquals("type", "vp")).size() > 0;
}
public boolean productExists(String product) {
return super.index.findDocuments(new IndexSearch().fieldEquals("product", product).fieldEquals("type", "pv")).size() > 0;
}
public Set findVendorsFuzzy(String vendor) {
return super.index.findDocuments(new IndexSearch().fieldContains("vendor", vendor).fieldEquals("type", "vp")).stream()
.map(d -> d.get("vendor"))
.collect(Collectors.toSet());
}
public Set findProductsFuzzy(String product) {
return super.index.findDocuments(new IndexSearch().fieldContains("product", product).fieldEquals("type", "pv")).stream()
.map(d -> d.get("product"))
.collect(Collectors.toSet());
}
public Set findVendorsForProduct(String product) {
return getProductVendorsMap().getOrDefault(product, Collections.emptySet());
}
public Set findProductsForVendor(String vendor) {
return getVendorProductsMap().getOrDefault(vendor, Collections.emptySet());
}
public Map> getVendorProductsMap() {
synchronized (vendorProductsMap) {
if (vendorProductsMap.size() == 0) {
LOG.debug("Building Vendor Products map for the first time");
super.index.findAndProcessAllDocuments(document -> vendorProductsMap.computeIfAbsent(document.get("vendor"), k -> new HashSet<>()).addAll(Arrays.asList(document.get("product").split(", "))));
}
}
return vendorProductsMap;
}
public Map> getProductVendorsMap() {
synchronized (productVendorsMap) {
if (productVendorsMap.size() == 0) {
LOG.debug("Building Product Vendors map for the first time");
super.index.findAndProcessAllDocuments(document -> productVendorsMap.computeIfAbsent(document.get("product"), k -> new HashSet<>()).addAll(Arrays.asList(document.get("vendor").split(", "))));
}
}
return productVendorsMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy