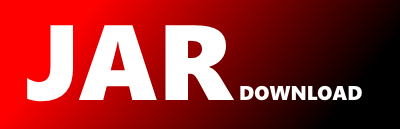
com.metaeffekt.mirror.query.MsrcAdvisorIndexQuery Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.mirror.query;
import com.metaeffekt.mirror.contents.advisory.MsrcAdvisorEntry;
import com.metaeffekt.mirror.contents.msrcdata.MsrcProduct;
import com.metaeffekt.mirror.index.IndexSearch;
import com.metaeffekt.mirror.index.advisor.MsrcAdvisorIndex;
import org.apache.lucene.document.Document;
import java.io.File;
import java.util.List;
import java.util.stream.Collectors;
public class MsrcAdvisorIndexQuery extends AdvisorIndexQuery {
public MsrcAdvisorIndexQuery(File baseMirrorDirectory) {
super(baseMirrorDirectory, MsrcAdvisorIndex.class);
}
public MsrcAdvisorIndexQuery(MsrcAdvisorIndex index) {
super(index);
}
@Override
public MsrcAdvisorEntry findById(String id) {
final boolean isAdv = id.contains("ADV");
id = id.replaceAll("(MSRC-)?(CVE|CAN)-?", "")
.replaceAll(".*?(\\d{1,4})-(\\d+).*", "$1-$2")
.replace("ADV", "");
id = isAdv ? "ADV" + id : "MSRC-CVE-" + id;
id = id.replaceAll("-+", "-");
return super.findById(id);
}
public List findByProduct(MsrcProduct product) {
return this.findByProduct(product.getId());
}
public List findByProduct(String productId) {
return super.index.findDocuments(new IndexSearch().fieldContains("affectedProducts", productId)).stream()
.map(this::createAdvisoryEntry)
.filter(e -> e.getAffectedProducts().contains(productId))
.collect(Collectors.toList());
}
/**
* The Ms Remediation entries sometimes contain a KB Identifier as their description. This method will find all
* {@link MsrcAdvisorEntry}s that have at least one {@link MsrcAdvisorEntry#getMsRemediations()} that contains this
* KB Identifier.
*
* This example contains the KB Id 5015807
:
*
* <vuln:Remediation Type="Vendor Fix">
* <vuln:Description>5015807</vuln:Description>
* <vuln:URL>https://catalog.update.microsoft.com/Error.aspx?id=-1914896368</vuln:URL>
* <vuln:Supercedence>5014699</vuln:Supercedence>
* <vuln:ProductID>11929</vuln:ProductID>
* <vuln:ProductID>11930</vuln:ProductID>
* <vuln:ProductID>11931</vuln:ProductID>
* <vuln:AffectedFiles/>
* <vuln:RestartRequired>Yes</vuln:RestartRequired>
* <vuln:SubType>Security Update</vuln:SubType>
* <vuln:FixedBuild>10.0.19044.1826</vuln:FixedBuild>
* </vuln:Remediation>
*
*
* @param kbIdentifier The KB Identifier to search for.
* @return A list of {@link MsrcAdvisorEntry}s that contain the KB Identifier.
*/
public List findAdvisorsByMsrcRemediationSupersededByKbId(String kbIdentifier) {
final String pureIdentifier = kbIdentifier.startsWith("KB") ? kbIdentifier.substring(2) : kbIdentifier;
return super.index.findDocuments(new IndexSearch().fieldContains("msRemediations", pureIdentifier)).stream()
.map(this::createAdvisoryEntry)
.filter(e -> e.getMsRemediations().stream().anyMatch(r -> r.getDescription().equals(pureIdentifier)))
.collect(Collectors.toList());
}
@Override
protected MsrcAdvisorEntry createAdvisoryEntry(Document document) {
return MsrcAdvisorEntry.fromDocument(document);
}
}