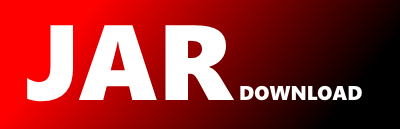
com.metaeffekt.resource.InventoryResource Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.resource;
import com.metaeffekt.artifact.analysis.utils.FileUtils;
import lombok.Getter;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.metaeffekt.core.inventory.processor.reader.InventoryReader;
import org.metaeffekt.core.inventory.processor.writer.InventoryWriter;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.util.LinkedHashMap;
import java.util.Map;
import static java.lang.String.format;
public class InventoryResource extends Resource {
private final static Logger LOG = LoggerFactory.getLogger(InventoryResource.class);
@Getter
private final File file;
private final Inventory providedInventory;
private Inventory loadedInventory;
public InventoryResource(File file, Inventory inventory) {
this.file = file;
this.providedInventory = inventory;
}
public Inventory getInventory() {
synchronized (this) {
if (loadedInventory == null) {
if (providedInventory != null) {
loadedInventory = providedInventory;
} else {
loadedInventory = reloadInventory();
}
}
}
return loadedInventory;
}
@Override
public void sync() throws IOException {
if (!file.exists()) {
FileUtils.forceMkdirParent(file);
}
new InventoryWriter().writeInventory(getInventory(), file);
}
@Override
public void sync(File targetFile) throws IOException {
if (targetFile.getParentFile() != null && !targetFile.getParentFile().exists()) {
FileUtils.forceMkdirParent(targetFile);
}
new InventoryWriter().writeInventory(getInventory(), targetFile);
}
public Map buildQualifierArtifactMap() {
final Inventory inventory = getInventory();
final Map qualifierArtifactMap = new LinkedHashMap<>();
for (final Artifact artifact : inventory.getArtifacts()) {
final String artifactQualifier = qualifierOf(artifact);
if (!qualifierArtifactMap.containsKey(artifactQualifier)) {
qualifierArtifactMap.put(artifactQualifier, artifact);
} else {
throw new IllegalStateException(String.format(
"Duplicate artifact qualifier detected in inventory [%s]. Qualifier: [%s]",
getFile(), artifactQualifier));
}
}
return qualifierArtifactMap;
}
public String qualifierOf(Artifact artifact) {
return "[" +
artifact.getId() + "-" +
artifact.getChecksum() + "-" +
artifact.getGroupId() + "-" +
artifact.getVersion() +
"]";
}
public Inventory reloadInventory() {
if (file != null && file.exists()) {
try {
return new InventoryReader().readInventory(file);
} catch (IOException e) {
throw new IllegalStateException("Cannot load inventory from file: [" + file.getAbsolutePath() + "]", e);
}
} else {
// when no file was given we return a new inventory
return new Inventory();
}
}
/**
* Creates the resource from the specified file. If the file does not exist an empty inventory is created. If
* The file already exists the inventory is loaded.
*
* @param inventoryFile The inventory file to be used
* @return The created inventory resource
*/
public static InventoryResource attachFile(File inventoryFile) {
return new InventoryResource(inventoryFile, null);
}
/**
* Initializes from an existing file. The file must exist.
*
* @param inventoryFile The inventory file to be used
* @return The created inventory resource
*/
public static InventoryResource fromFile(File inventoryFile) {
validateInventoryFile(inventoryFile);
return new InventoryResource(inventoryFile, null);
}
private static void validateInventoryFile(File inventoryFile) {
if (!inventoryFile.exists()) {
throw new IllegalStateException(String.format("Inventory file [%s] does not exist.", inventoryFile));
}
}
public static InventoryResource fromInventory(Inventory inventory) {
return new InventoryResource(null, inventory);
}
public static InventoryResource create() {
return new InventoryResource(null, null);
}
public void syncIfPossible(File inventoryFile) {
try {
sync(inventoryFile);
} catch (IOException e) {
LOG.warn(format("Cannot write inventory from [%s].", inventoryFile));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy