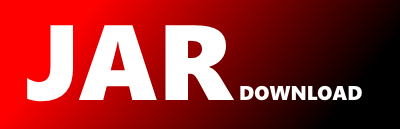
com.metaeffekt.artifact.analysis.flow.SpdxDocumentFlow Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.flow;
import com.metaeffekt.artifact.analysis.bom.BomConstants;
import com.metaeffekt.artifact.analysis.bom.spdx.DocumentSpec;
import com.metaeffekt.artifact.analysis.bom.spdx.SpdxExporter;
import com.metaeffekt.artifact.analysis.model.PropertyProvider;
import com.metaeffekt.artifact.analysis.utils.FileUtils;
import com.metaeffekt.artifact.terms.model.LicenseTextProvider;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import com.metaeffekt.resource.InventoryResource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.util.UUID;
public class SpdxDocumentFlow {
private static final Logger LOG = LoggerFactory.getLogger(SpdxDocumentFlow.class);
public void process(SpdxDocumentFlowParam spdxDocumentFlowParam) throws IOException {
// instantiate exporter
final SpdxExporter spdxExporter =
new SpdxExporter(null,
spdxDocumentFlowParam.getNormalizationMetaData(), spdxDocumentFlowParam.getLicenseTextProvider(), null);
// produce spdx document
try {
spdxExporter.exportToSpdxDocument(
spdxDocumentFlowParam.getInventoryResource().getInventory(),
spdxDocumentFlowParam.getTargetSpdxFile(),
spdxDocumentFlowParam.getDocumentSpec(),
true);
} catch (RuntimeException e) {
LOG.warn("Cannot produce SPDX document [{}]", spdxDocumentFlowParam.getTargetSpdxFile(), e);
}
}
public static void produceSpdxDocument(InventoryResource inventoryResource, String name, File targetSpdxDocumentFile,
NormalizationMetaData normalizationMetaData, LicenseTextProvider licenseTextProvider,
PropertyProvider propertyProvider) throws IOException {
FileUtils.forceMkdirParent(targetSpdxDocumentFile);
final String organization = propertyProvider.getProperty("spdx.document.organization", null);
final String person = propertyProvider.getProperty("spdx.document.person", null);
final String tool = propertyProvider.getProperty("spdx.document.tool", "metaeffekt Kontinuum SPDX Exporter");
final String uriPrefix = propertyProvider.getProperty("spdx.document.uri.prefix", null);
final String description = propertyProvider.getProperty("spdx.document.description", null);
final String comment = propertyProvider.getProperty("spdx.document.comment", null);
final String spdxLicenseListVersion = propertyProvider.getProperty("spdx.document.license.list.version", null);
final String organizationUrl = propertyProvider.getProperty("spdx.document.organization.url", null);
final DocumentSpec documentSpec = new DocumentSpec(name,
uriPrefix + UUID.randomUUID(), organization, organizationUrl)
.withDescription(description)
.withComment(comment)
.withSpdxLicenseListVersion(spdxLicenseListVersion)
.byPerson(person)
.usingTool(tool)
.usingFormat(BomConstants.Format.JSON);
final SpdxDocumentFlowParam spdxDocumentFlowParam =
SpdxDocumentFlowParam.build()
.using(inventoryResource)
.using(normalizationMetaData)
.using(licenseTextProvider)
.documenting(documentSpec)
.writing(targetSpdxDocumentFile);
new SpdxDocumentFlow().process(spdxDocumentFlowParam);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy