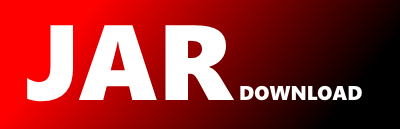
com.metaeffekt.artifact.analysis.flow.ng.TermMetaDataProducer Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.flow.ng;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.param.NormMetaSupplierParameters;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.param.ProviderParameters;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.tmd.EncryptedTermMetaDataProviderNG;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.tmd.EncryptedTermMetaDataSupplierNG;
import com.metaeffekt.artifact.analysis.flow.ng.keyholder.UserKeysForConsumer;
import com.metaeffekt.artifact.analysis.flow.ng.keyholder.UserKeysForSupplier;
import com.metaeffekt.artifact.analysis.preprocess.filter.wordlist.WordlistGenerator;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang3.tuple.Pair;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.nio.file.Files;
import java.security.SecureRandom;
import java.util.Collection;
import java.util.List;
import static com.metaeffekt.artifact.analysis.flow.ng.EncryptedArtifactProducerUtils.*;
/**
* Helper for producing encrypted tmd objects.
* Handles some required preparation and setup.
* Takes care of filtering customer metadata before passing {@link NormalizationMetaData} with
* {@link EncryptedArtifactProducerUtils#filterCustomerMetaData(NormalizationMetaData)}.
*/
public class TermMetaDataProducer {
private static final Logger LOG = LoggerFactory.getLogger(TermMetaDataProducer.class);
/**
* Produces an encrypted {@link NormalizationMetaData} object using the given spec
* (and data in the file system as referenced by the spec and such).
*
* @param spec containing parameters used in the process.
* @throws Exception unlikely the exceptions thrown here could be remedied in code, honestly. Rethrowing for logs.
*/
public static void produce(TermMetaDataProducerSpec spec) throws Exception {
// temp dir for temporary keys. unused if test is disabled
File tempDir = Files.createTempDirectory("tmdGenerate-tempKeys-").toFile();
FileUtils.forceDeleteOnExit(tempDir);
final File dummyDecryptionKeysDir = new File(tempDir, "decryptionTestKeys");
final File allowedKeysWithDummy = new File(tempDir, "allowedKeys");
final File dummyZipOutputFile = new File(tempDir, "terms-metadata");
final SecureRandom random = new SecureRandom();
File dummyKeyForUser = null;
String temporaryPassword = null;
if (spec.isEnableImplicitTest()) {
// initialize a dummy access key for testing
Pair keypair = KeypairGenerator.createUserKeyPair(random);
UserKeysForSupplier keysForSupplier = keypair.getLeft();
UserKeysForConsumer keysForConsumer = keypair.getRight();
File dummyKeyForPublisher = new File(allowedKeysWithDummy, "dummyKeyForPublisher.tmp.json");
dummyKeyForUser = new File(dummyDecryptionKeysDir, "dummyKeyForUser.tmp.json");
temporaryPassword = KeypairGenerator.generateUserKeysPassword(64);
// write the generated dummy key
writeDummyKeys(dummyKeyForPublisher, dummyKeyForUser, keysForSupplier, keysForConsumer, temporaryPassword);
}
if (dummyKeyForUser == null) {
throw new IllegalStateException();
}
// read terms metadata
final NormalizationMetaData normalizationMetaData = new NormalizationMetaData(spec.getTermsMetaDataBaseDir());
// explicitly generate wordlist
normalizationMetaData.generateAndSetWordlist();
// filter customer related information unless the build is for internal use only
if (spec.getBuildType() != TermMetaDataProducerSpec.BuildType.INTERNAL) {
LOG.debug("Filtering customer metadata.");
filterCustomerMetaData(normalizationMetaData);
} else {
LOG.info("Skipping data filtering for type [{}]. Will include customer licenses.", spec.getBuildType());
}
// prepare for encryption
final NormMetaSupplierParameters supplierNGParam = new NormMetaSupplierParameters(
new ContentAlgorithmParam(),
normalizationMetaData,
spec.getAllowedUserKeysDir(),
spec.getLicenseTextFile(),
spec.getZipOutputFile()
);
LOG.info("Beginning output of encrypted content.");
// encrypt to blob and write keyslots file
final EncryptedTermMetaDataSupplierNG supplier = new EncryptedTermMetaDataSupplierNG();
supplier.process(supplierNGParam);
if (spec.isEnableImplicitTest()) {
LOG.info("Beginning test with dummy key.");
// separate encryption run with the same data but an additional dummy key
final NormMetaSupplierParameters dummySupplierParam = new NormMetaSupplierParameters(
new ContentAlgorithmParam(),
normalizationMetaData,
allowedKeysWithDummy,
spec.getLicenseTextFile(),
dummyZipOutputFile
);
// encrypt to blob and write keyslots file
final EncryptedTermMetaDataSupplierNG dummySupplier = new EncryptedTermMetaDataSupplierNG();
dummySupplier.process(dummySupplierParam);
// decryption crash test
ProviderParameters providerParameters = new ProviderParameters(
new ContentAlgorithmParam(),
temporaryPassword,
dummyKeyForUser,
dummyZipOutputFile);
// decrypt blob for testing
final EncryptedTermMetaDataProviderNG provider = new EncryptedTermMetaDataProviderNG();
NormalizationMetaData readNormMeta = provider.process(providerParameters);
// check metadata for equality
assertEquals(normalizationMetaData.getLicenseNameMap(), readNormMeta.getLicenseNameMap());
assertEquals(normalizationMetaData.getLicenseMetaDataMap(), readNormMeta.getLicenseMetaDataMap());
assertEquals(normalizationMetaData.getCategoryNameMap(), readNormMeta.getCategoryNameMap());
// generate wordlist again for testing
Collection generatedWordlist = WordlistGenerator.createWordlist(normalizationMetaData);
assertEquals(readNormMeta.getWordlist().size(), generatedWordlist.size());
assertWordlistEquals(readNormMeta.getWordlist(), generatedWordlist);
// apply original and serialized
final List analyzeRead = readNormMeta.analyze("This targetContentFile is under the Apache License 2.0.");
final List analyzeExpected = normalizationMetaData.analyze("This targetContentFile is under the Apache License 2.0.");
// check results are identical
assertEquals(analyzeExpected, analyzeRead);
// dump analysis result
LOG.info("Analysis result: " + analyzeRead);
LOG.info("Wordlist size: " + (readNormMeta.getWordlist() == null ? "n.a." : readNormMeta.getWordlist().size()));
} else {
LOG.warn("Implicit output testing is disabled!");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy