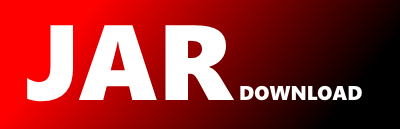
com.metaeffekt.artifact.analysis.flow.ng.crypt.DecryptedEntryInputStream Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.flow.ng.crypt;
import com.metaeffekt.artifact.analysis.flow.ng.ContentAlgorithmParam;
import org.apache.commons.io.input.CloseShieldInputStream;
import javax.crypto.AEADBadTagException;
import javax.crypto.Cipher;
import javax.crypto.CipherInputStream;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.spec.IvParameterSpec;
import java.io.FilterInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.security.*;
import java.util.zip.ZipInputStream;
import static com.metaeffekt.artifact.analysis.flow.ng.StreamReadingUtils.readOrThrow;
/**
* Useful for reading zip entries encrypted by {@link EncryptedEntryOutputStream}.
*/
public class DecryptedEntryInputStream extends FilterInputStream implements AutoCloseable {
private final boolean closeUnderlying;
private boolean closed = false;
/**
* Stored only so we can cleanly run closeEntry at the end.
*/
private final ZipInputStream underlyingZipStream;
private DecryptedEntryInputStream(ZipInputStream underlyingZipStream, Cipher cipher, boolean closeUnderlying) {
// wrap the underlying stream to encrypt this entry
super(new CipherInputStream(CloseShieldInputStream.wrap(underlyingZipStream), cipher));
this.underlyingZipStream = underlyingZipStream;
this.closeUnderlying = closeUnderlying;
}
private static Cipher createDecryptionCipher(ContentAlgorithmParam param, Key key, byte[] iv)
throws NoSuchAlgorithmException, InvalidKeyException, NoSuchProviderException, NoSuchPaddingException, InvalidAlgorithmParameterException {
Cipher cipher = Cipher.getInstance(param.getAlgorithm() + param.getMode(), param.getProvider());
cipher.init(Cipher.DECRYPT_MODE, key, new IvParameterSpec(iv));
return cipher;
}
public static DecryptedEntryInputStream createEntryDecryptionInputStream(ZipInputStream zipInputStream,
ContentAlgorithmParam algorithmParam,
Key contentKey)
throws InvalidAlgorithmParameterException, NoSuchPaddingException, NoSuchAlgorithmException,
NoSuchProviderException, InvalidKeyException, IOException {
return createEntryDecryptionInputStream(zipInputStream, algorithmParam, contentKey, false);
}
public static DecryptedEntryInputStream createEntryDecryptionInputStream(ZipInputStream zipInputStream,
ContentAlgorithmParam algorithmParam,
Key contentKey,
boolean closeUnderlying)
throws IOException, InvalidAlgorithmParameterException, NoSuchPaddingException, NoSuchAlgorithmException,
InvalidKeyException, NoSuchProviderException {
// the nonce needs separate transmission but NOT in AAD thanks to EAX's guarantees
byte[] iv = new byte[16];
// read iv from the beginning of the current entry
readOrThrow(zipInputStream, iv);
Cipher cipher = createDecryptionCipher(algorithmParam, contentKey, iv);
return new DecryptedEntryInputStream(zipInputStream, cipher, closeUnderlying);
}
public static void ensureAuthenticated(InputStream zipInputStream,
ContentAlgorithmParam param,
Key contentKey) throws AEADBadTagException {
try {
// the nonce needs separate transmission but NOT in AAD thanks to EAX's guarantees
byte[] iv = new byte[16];
// read iv from the beginning of the current entry
readOrThrow(zipInputStream, iv);
Cipher cipher = createDecryptionCipher(param, contentKey, iv);
byte[] buffer = new byte[4096];
while (true) {
int readBytes = zipInputStream.read(buffer);
if (readBytes == -1) {
break;
} else if (readBytes > 0) {
cipher.update(buffer, 0, readBytes);
}
}
// throws if not authenticated
cipher.doFinal();
} catch (AEADBadTagException e) {
throw e;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* Closes the current entry in the underlying ZipOutputStream but DOES NOT close the stream.
*/
@Override
public void close() throws IOException{
// make it possible to use these in try-with-resources to avoid unclosed entries
if (!this.closed) {
this.closed = true;
// closes the cipher stream
super.close();
// closing this helper object must close the zip entry
underlyingZipStream.closeEntry();
if (closeUnderlying) {
// only close the underlying stream if it's actually wanted
underlyingZipStream.close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy