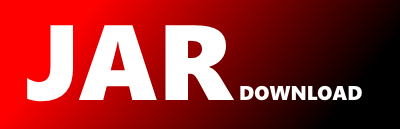
com.metaeffekt.artifact.analysis.flow.ng.crypt.tmd.EncryptedTermMetaDataProviderNG Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.flow.ng.crypt.tmd;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.EncryptedZipProvider;
import com.metaeffekt.artifact.analysis.flow.ng.crypt.param.ProviderParameters;
import com.metaeffekt.artifact.analysis.utils.InventoryUtils;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import com.metaeffekt.flow.common.AbstractFlow;
import org.bouncycastle.crypto.InvalidCipherTextException;
import javax.crypto.NoSuchPaddingException;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.util.zip.GZIPInputStream;
import static com.metaeffekt.artifact.analysis.flow.ng.EncryptedArchiveConstants.DATASET_ENTRY_NAME;
/**
* Reads and returns a {@link NormalizationMetaData} object from a suitable encrypted zip.
*/
public class EncryptedTermMetaDataProviderNG extends AbstractFlow {
public NormalizationMetaData process(ProviderParameters param) {
try {
return readTermMetaData(param);
} catch (Exception e) {
throw new RuntimeException(
"An odd Exception likely indicates misconfiguration (for example): " +
"dataset / scanner version mismatch, " +
"keys unauthorized for this artifact, key or data integrity error, " +
"invalid dataset or keyfile locations, (or other). " +
"Please contact {metaeffekt} for support.", e
);
}
}
/**
* Decrypts the content file (from zip) using the content key.
* @param param params to read the zip's location and algorithm parameters from
* @return returns decrypted norm meta
* @throws IOException throws on io error
* @throws InvalidAlgorithmParameterException throws on decryption error
* @throws NoSuchPaddingException throws on decryption error
* @throws NoSuchAlgorithmException throws on decryption error
* @throws InvalidKeyException throws on decryption error
* @throws NoSuchProviderException throws on decryption error
*/
private NormalizationMetaData readContentFile(ProviderParameters param)
throws IOException,
InvalidAlgorithmParameterException,
NoSuchPaddingException,
NoSuchAlgorithmException,
InvalidKeyException,
NoSuchProviderException,
ClassNotFoundException,
InvalidCipherTextException {
EncryptedZipProvider provider = new EncryptedZipProvider(param);
try (InputStream inputStream = provider.getEntryStream(DATASET_ENTRY_NAME);
final GZIPInputStream gzipInputStream = new GZIPInputStream(inputStream);
final ObjectInputStream objectInputStream = new ObjectInputStream(gzipInputStream)) {
// read the encoded object
final NormalizationMetaData normalizationMetaData = (NormalizationMetaData) objectInputStream.readObject();
// FIXME: static reference
InventoryUtils.initialize(normalizationMetaData);
return normalizationMetaData;
}
}
protected NormalizationMetaData readTermMetaData(ProviderParameters param)
throws NoSuchProviderException, NoSuchPaddingException, NoSuchAlgorithmException, InvalidKeyException, IOException, ClassNotFoundException, InvalidAlgorithmParameterException, InvalidCipherTextException {
// now read the content file
return readContentFile(param);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy