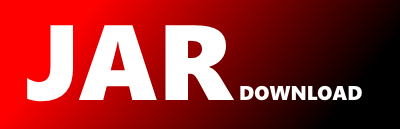
com.metaeffekt.artifact.analysis.workbench.InventoryTransformer Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.workbench;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import org.apache.commons.lang.StringUtils;
import org.metaeffekt.common.notice.model.ComponentDefinition;
import org.metaeffekt.common.notice.model.NoticeParameters;
import org.metaeffekt.core.inventory.InventoryUtils;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.metaeffekt.notice.NoticeParameterProcessor;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
import java.util.stream.Collectors;
public class InventoryTransformer {
private static final Logger LOG = LoggerFactory.getLogger(InventoryTransformer.class);
private final static String NOTICE_PARAMETER = "Notice Parameter";
private Set reportedLicenses = new HashSet<>();
private final NormalizationMetaData normalizationMetaData;
public InventoryTransformer(NormalizationMetaData normalizationMetaData) {
this.normalizationMetaData = normalizationMetaData;
}
public Inventory updateCanonicalNamesInInventory(final Inventory inventory) {
if (inventory != null) {
updateLicensesInInventory(inventory);
updateLicensesInNoticeParam(inventory);
}
return inventory;
}
private Inventory updateLicensesInInventory(final Inventory inventory) {
for (Artifact artifact : inventory.getArtifacts()) {
updateLicense(artifact);
}
return inventory;
}
private void updateLicense(final Artifact artifact) {
final List updatedCanonicalNames = updateLicenses(artifact.getLicenses());
artifact.setLicense(InventoryUtils.joinLicenses(updatedCanonicalNames));
}
private Inventory updateLicensesInNoticeParam(Inventory inventory) {
// NoticeParameterProcessor is stateless and can be reused for all notices
final NoticeParameterProcessor noticeParameterProcessor = new NoticeParameterProcessor(null);
for (Artifact artifact : inventory.getArtifacts()) {
try {
if (artifact.get(NOTICE_PARAMETER) != null) {
NoticeParameters param = noticeParameterProcessor.readNoticeParameter(artifact.get(NOTICE_PARAMETER));
updateNoticeParameter(param);
artifact.set(NOTICE_PARAMETER, param.toYamlString());
}
} catch (Exception e) {
LOG.warn("Cannot process notice parameter for artifact {}.", artifact.deriveQualifier(), e);
}
}
return inventory;
}
public void updateNoticeParameter(NoticeParameters param) {
// component
updateComponentDefinition(param.getComponent());
// subcomponents
if (param.getSubcomponents() != null) {
for (ComponentDefinition subcomponent : param.getSubcomponents()) {
updateComponentDefinition(subcomponent);
}
}
}
private void updateComponentDefinition(ComponentDefinition component) {
// validation is not performed here; just skip null component definition
if (component == null) return;
component.setAssociatedLicenses(updateLicenses(component.getAssociatedLicenses()));
final List effectiveLicenses = component.getEffectiveLicenses();
if (effectiveLicenses != null && !effectiveLicenses.isEmpty()) {
component.setEffectiveLicenses(updateLicenses(effectiveLicenses));
}
}
public List updateLicenses(Collection licenseList) {
final List updatedCanonicalNames = new ArrayList<>();
for (String license : licenseList) {
// and tokenize
for (String tokenizedLicense : InventoryUtils.tokenizeLicense(license, false, true)) {
// or tokenize
List multiLicense = new ArrayList<>();
for (String atomicLicense : InventoryUtils.tokenizeLicense(tokenizedLicense, false, false)) {
multiLicense.add(getUpdatedCanonicalName(atomicLicense));
}
// ... and joined again with the + separator
final String resultLicenseExpression = StringUtils.join(multiLicense, " + ");
updatedCanonicalNames.add(resultLicenseExpression);
}
}
return updatedCanonicalNames;
}
public String transformLicenseExpression(String licenseExpression) {
// 'and' tokenize
final List licenseList = InventoryUtils.tokenizeLicense(licenseExpression, false, true);
return InventoryUtils.joinLicenses(transformLicenses(licenseList));
}
public List transformLicenses(List licenseList) {
final List collectedSubExpressions = new ArrayList<>();
for (String tokenizedLicenseExpression : licenseList) {
// 'or' tokenize
Set multiLicense = new LinkedHashSet<>();
for (String atomicLicense : InventoryUtils.tokenizeLicense(tokenizedLicenseExpression, false, false)) {
multiLicense.add(getUpdatedCanonicalName(atomicLicense));
}
// ... and joined again with the + separator
final String resultLicenseExpression = StringUtils.join(multiLicense, " + ");
collectedSubExpressions.add(resultLicenseExpression);
}
return collectedSubExpressions.stream().collect(Collectors.toList());
}
public String getUpdatedCanonicalName(String license) {
return normalizationMetaData.getUpdatedCanonicalName(license);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy