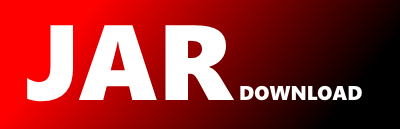
com.metaeffekt.artifact.analysis.workbench.LicenseAggregationUtils Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metaeffekt.artifact.analysis.workbench;
import com.metaeffekt.artifact.analysis.utils.FileUtils;
import com.metaeffekt.artifact.analysis.utils.InventoryUtils;
import com.metaeffekt.artifact.terms.model.LicenseTextEntry;
import com.metaeffekt.artifact.terms.model.LicenseTextProvider;
import com.metaeffekt.artifact.terms.model.NormalizationMetaData;
import com.metaeffekt.artifact.terms.model.TermsMetaData;
import com.metaeffekt.resource.InventoryResource;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Inventory;
import org.metaeffekt.core.inventory.processor.model.LicenseData;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.util.*;
import static org.metaeffekt.core.inventory.InventoryUtils.tokenizeLicense;
public class LicenseAggregationUtils {
private static final Logger LOG = LoggerFactory.getLogger(LicenseAggregationUtils.class);
public static List copyLicenses(Inventory inventory, File targetProjectDir,
NormalizationMetaData normalizationMetaData, LicenseTextProvider licenseTextProvider, boolean failOnError) {
final boolean filterForOpenCode = false;
final File targetProjectLicencesDir = new File(targetProjectDir, "licenses");
final Set allLicenses = new HashSet<>();
// collect associated and effective licenses
for (Artifact artifact : inventory.getArtifacts()) {
final String associatedLicense = artifact.getLicense();
final String effectiveLicense = inventory.getEffectiveLicense(artifact);
allLicenses.addAll(tokenizeLicense(associatedLicense, false, true));
allLicenses.addAll(tokenizeLicense(effectiveLicense, false, false));
}
// preserve current inventory license data by adding the licenses as they exist
for (final LicenseData licenseData : inventory.getLicenseData()) {
allLicenses.add(licenseData.get(LicenseData.Attribute.CANONICAL_NAME));
}
final List collectedCanonicalNames = new ArrayList<>();
final InventoryTransformer inventoryTransformer = new InventoryTransformer(normalizationMetaData);
final Map licenseToNormalizedMetaDataMap = new HashMap<>();
for (String licenseExpression : allLicenses) {
for (String license : InventoryUtils.tokenizeLicense(licenseExpression, false, false)) {
String normalizedLicense = license.trim();
// apply canonical name updates using inventory transformer
normalizedLicense = inventoryTransformer.getUpdatedCanonicalName(normalizedLicense);
TermsMetaData termsMetaData = normalizationMetaData.getTermsMetaData(normalizedLicense);
// honor named equivalence (in case no text is supplied)
if (termsMetaData != null) {
final String namedEquivalence = termsMetaData.getNamedEquivalence();
if (namedEquivalence != null) {
termsMetaData = normalizationMetaData.getTermsMetaData(namedEquivalence);
normalizedLicense = namedEquivalence;
}
}
// FIXME: consider other types of license relationships; e.g. baseTerms
// memorize mapping from original license to TMD
licenseToNormalizedMetaDataMap.put(normalizedLicense, termsMetaData);
licenseToNormalizedMetaDataMap.putIfAbsent(license, termsMetaData);
}
}
// resolve license text using provider
// FIXME: licenseTextEntry should also support readmes.
final Map licenseTextMap = licenseTextProvider.resolve(licenseToNormalizedMetaDataMap.keySet());
for (Map.Entry licenseMapEntry : licenseToNormalizedMetaDataMap.entrySet()) {
final String licenseExpression = licenseMapEntry.getKey();
for (String license : InventoryUtils.tokenizeLicense(licenseExpression, false, false)) {
final File targetDir = new File(targetProjectLicencesDir, inventory.getLicenseFolder(license));
final TermsMetaData termsMetaData = licenseToNormalizedMetaDataMap.get(license);
if (termsMetaData == null) {
final String message = "No license metadata available, cannot copy license. Normalized license: [" + licenseExpression + "]; license: [" + license + "]";
if (failOnError) {
throw new IllegalStateException(message);
} else {
LOG.warn(message);
}
} else {
LicenseTextEntry licenseFile = licenseTextMap.get(licenseExpression);
if (licenseFile == null) {
if (termsMetaData.isExpression() || termsMetaData.isMarker() || termsMetaData.isUnspecific()) continue;
// fallback to readme
if (!filterForOpenCode) {
// FIXME: LicenseTextEntry should also support a readme.
// licenseFile = termsMetaData.getReadmeFile();
}
}
if (licenseFile != null) {
try {
final File targetFile = new File(targetDir, new File(licenseFile.getFilename()).getName());
FileUtils.write(targetFile, licenseFile.getLicenseText(), FileUtils.ENCODING_UTF_8);
} catch (IOException e) {
if (failOnError) {
throw new IllegalStateException("Cannot copy license files.", e);
}
}
} else {
if (!termsMetaData.isExpression()) {
final String message = "No license file available for [" + license + "]";
if (failOnError) {
throw new IllegalStateException(message);
} else {
System.out.println(message);
}
continue;
}
}
long copiedFiles = targetDir.exists() ? FileUtils.scanDirectoryForFiles(targetDir, true, "*.*").length : 0;
if (copiedFiles == 0) {
if (failOnError) {
throw new IllegalStateException("No license file provided for: " + license);
}
}
}
}
}
return collectedCanonicalNames;
}
public static void fillLicenseMetaData(InventoryResource inventoryResource, NormalizationMetaData normalizationMetaData) {
for (LicenseData licenseData : inventoryResource.getInventory().getLicenseData()) {
final String canonicalName = licenseData.get(LicenseData.Attribute.CANONICAL_NAME);
TermsMetaData termsMetaData = normalizationMetaData.getTermsMetaData(canonicalName);
if (termsMetaData == null) {
termsMetaData = normalizationMetaData.findUsingCanonicalNameInHistory(canonicalName);
}
if (termsMetaData != null) {
InventoryUtils.transferTmdAttributesToLicenseData(licenseData, termsMetaData);
// adjust canonical name; track origin
if (!canonicalName.equals(termsMetaData.getCanonicalName())) {
licenseData.set(LicenseData.Attribute.CANONICAL_NAME, termsMetaData.getCanonicalName());
licenseData.set("Original Canonical Name", canonicalName);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy