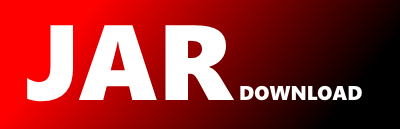
org.metaeffekt.artifact.resolver.alpine.bodge.StringPieceAssembler Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.alpine.bodge;
import lombok.Getter;
import lombok.NonNull;
import lombok.Setter;
import lombok.extern.slf4j.Slf4j;
import org.metaeffekt.artifact.resolver.alpine.AlpinePackageReference;
import java.util.List;
import java.util.Objects;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Processs a list of {@link StringPiece} to create a full download url.
*/
@Getter
@Setter
@Slf4j
public class StringPieceAssembler {
protected static String getRefValue(@NonNull AlpinePackageReference packageRef,
@NonNull StringPiece.RefValue refValue) {
switch (refValue) {
case PKGREL:
return packageRef.getPkgrel();
case PKGVER:
return packageRef.getPkgver();
case ALPINE_VERSION:
return packageRef.getAlpineVersion();
default:
throw new IllegalArgumentException("Unknown / unimplemented refValue given.");
}
}
/**
* Runs full derivation of a piece of string from the given specification.
* @param piece the piece to run derivation on
* @param packageRef package reference to get (meta) data from
* @return the result of all processing (concatenated: (literal + processed) in case both are defined)
*/
public static String runDerivationForPiece(@NonNull StringPiece piece, @NonNull AlpinePackageReference packageRef) {
final StringBuilder urlBuilder = new StringBuilder();
urlBuilder.append(piece.getLiteral());
// stop here if further definition is incomplete
if (piece.getFromValue() == null) {
return urlBuilder.toString();
}
// string to be processed
String inProcessing = getRefValue(packageRef, piece.getFromValue());
if (piece.getActions() != null) {
for (StringAction action : piece.getActions()) {
switch (action.getActionType()) {
case PLAIN_REPLACEALL:
Objects.requireNonNull(action.getFrom(), "String piece action " + action.getActionType() +
" requires non-null to and from.");
Objects.requireNonNull(action.getTo(), "String piece action " + action.getActionType() +
" requires non-null to and from.");
inProcessing = inProcessing.replaceAll(Pattern.quote(action.getFrom()), action.getTo());
break;
default:
log.error("Unsupported action [{}].", action.getActionType());
throw new IllegalArgumentException("Action not supported by this version of StringPieceAssembler.");
}
}
}
// pattern for final matching
final Pattern finalMatchPattern = Pattern.compile(piece.getFinallyMatch());
final Matcher finalMatcher = finalMatchPattern.matcher(inProcessing);
if (finalMatcher.find()) {
// return this match
urlBuilder.append(finalMatcher.group());
} else {
log.warn(
"URL creation on package ref [{}] with urlPiece [{}] failed matching [{}] with pattern [{}].",
packageRef,
piece,
inProcessing,
piece.getFinallyMatch()
);
// do nothing. just warn. we won't append to urlBuilder since the match is empty
}
return urlBuilder.toString();
}
public static String createString(List pieces, AlpinePackageReference packageRef) {
StringBuilder urlBuilder = new StringBuilder();
for (StringPiece piece : pieces) {
urlBuilder.append(runDerivationForPiece(piece, packageRef));
}
return urlBuilder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy