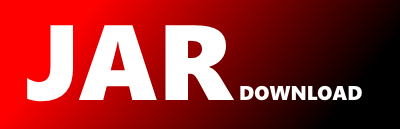
org.metaeffekt.artifact.resolver.deb.DebArtifactReference Maven / Gradle / Ivy
The newest version!
package org.metaeffekt.artifact.resolver.deb;
import com.github.packageurl.PackageURL;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.metaeffekt.artifact.resolver.generic.FileLocation;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
@Getter
@Slf4j
public class DebArtifactReference {
/**
* Name of the pacakge.
* For example. this is called "Package" in control files, "Source" in source files.
*/
private String name = null;
/**
* Version of this package.
* {@code [epoch:]upstream_version[-debian_revision]} -
*
* https://debian.org/doc/...
*/
private String version = null;
/**
* Architecture field as defined in debian control files. This means it can also point out "source".
*/
private String architecture = null;
/**
* Namespace of the package. According to the purl doc, this is supposed to identify the vendor.
*
* Most notably "debian" and "ubuntu".
*/
private DebNamespace namespace = null;
/**
* Enum for supported namespaces.
*
* Being unsupported means a null reference (enforced by constructors, in line with other reference impls).
*/
public enum DebNamespace {
DEBIAN,
UBUNTU;
}
public DebArtifactReference(PackageURL purl) {
if (!"deb".equals(purl.getType())) {
log.error("Purl [{}] isn't a deb purl.", purl);
return;
}
final String name = purl.getName();
final String version = purl.getVersion();
if (name != null && name.contains(" ")) {
log.warn("Given purl [{}] contains space in name. This is not allowed. Aborting resolution.",
purl);
// return without setting any fields to make this object invalid
return;
}
if (version != null && version.contains(" ")) {
log.warn("Given purl [{}] contains space in version. This is not allowed. Aborting resolution.",
purl);
// return without setting any fields to make this object invalid
return;
}
final Map qualifiers = purl.getQualifiers() == null ? new HashMap<>() : purl.getQualifiers();
final String architecture = qualifiers.get("arch");
DebNamespace namespace = null;
try {
namespace = purl.getNamespace() == null ?
null :
DebNamespace.valueOf(purl.getNamespace().toUpperCase(Locale.ENGLISH));
} catch (IllegalArgumentException e) {
log.trace("Rejected purl [{}] of incompatible namespace.", purl);
}
if (name != null && version != null && namespace != null) {
this.name = name;
this.version = version;
this.architecture = architecture;
this.namespace = namespace;
} else {
log.trace("One of name, version, namespace was null: Invalidating due to params [{}], [{}], [{}].",
name,
version,
namespace);
}
}
public DebArtifactReference(String name, String version, String architecture, DebNamespace namespace) {
if (name != null && version != null && namespace != null) {
this.name = name;
this.version = version;
this.architecture = architecture;
this.namespace = namespace;
}
}
public FileLocation deriveFileLocation(String filename) {
FileLocation fileLocation = new FileLocation();
fileLocation.setEcosystem("deb-" + this.getNamespace().toString().toLowerCase(Locale.ENGLISH));
fileLocation.setFilename(filename);
fileLocation.setDispatchName(this.getName());
fileLocation.setResourceQualifier(this.getNamespace().toString() + "-" +
this.getName() + "_" + this.getVersion() + "_" + getArchitecture());
if (!fileLocation.isValid()) {
throw new IllegalStateException("File location invalid: " + fileLocation);
}
return fileLocation;
}
public boolean isValid() {
return StringUtils.isNotBlank(this.name) &&
StringUtils.isNotBlank(this.version) &&
StringUtils.isNotBlank(this.architecture) &&
this.namespace != null;
}
@Override
public String toString() {
return "DebArtifactReference{" +
"name='" + name + '\'' +
", version='" + version + '\'' +
", architecture='" + architecture + '\'' +
", namespace=" + namespace +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy