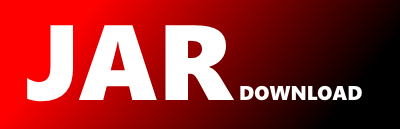
org.metaeffekt.artifact.resolver.generic.AbstractDownloadingAdapter Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.generic;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.HttpResponse;
import org.metaeffekt.artifact.resolver.download.WebAccess;
import org.metaeffekt.artifact.resolver.generic.utils.MarkerUtils;
import org.metaeffekt.artifact.resolver.model.DownloadLocation;
import org.metaeffekt.core.util.FileUtils;
import java.io.File;
import java.io.IOException;
import java.util.function.Consumer;
@Getter
@Slf4j
public abstract class AbstractDownloadingAdapter extends AbstractAdapter {
private final DownloadLocation downloadLocation;
private final WebAccess webAccess;
public AbstractDownloadingAdapter(DownloadLocation downloadLocation, WebAccess webAccess) {
this.downloadLocation = downloadLocation;
this.webAccess = webAccess;
}
protected void removeMarker(File markerFile) {
if (markerFile.exists()) {
FileUtils.deleteQuietly(markerFile);
}
}
/**
* Note that notions of how "failure" is written to the marker file differ across the codebase.
*
* Simply checks if the marker was modified recently, thus matching the old behaviour.
* @param markerFile the file to check
* @return whether a download was attempted recently (i.e. whether the file was modified "recently")
*/
protected boolean hasRecentFailedDownloadAttempt(File markerFile) {
return MarkerUtils.hasRecentDownloadAttempt(markerFile);
}
public File deriveDownloadFile(FileLocation fileLocation) {
final File resourceFolder = deriveResourceDir(fileLocation);
return new File(resourceFolder, fileLocation.getFilename());
}
protected File deriveMarkerFile(FileLocation fileLocation, String markerDescriminator) {
final File resourceDir = deriveResourceDir(fileLocation);
final File markerDir = new File(resourceDir, "MARKER");
final File repoMarkerFile = new File(markerDir, "[" + markerDescriminator.replaceAll("[/:]+", "_") + "]");
return repoMarkerFile;
}
protected File deriveResourceDir(FileLocation fileLocation) {
final File downloadDir = downloadLocation.deriveDownloadFolder(
fileLocation.getEcosystem(), fileLocation.getDispatchName());
final File resourceFolder = new File(downloadDir, "[" + fileLocation.getResourceQualifier() + "]");
return resourceFolder;
}
protected Consumer manageMarkerErrorResponseConsumer(final File markerFile) {
return httpResponse -> {
try {
FileUtils.forceMkdirParent(markerFile);
if (!markerFile.createNewFile()) {
log.warn("Could not create marker file [{}].", markerFile);
}
} catch (IOException e) {
// unmanaged marker file is not critical
log.warn("Could not manage marker file [{}].", markerFile);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy