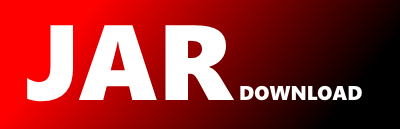
org.metaeffekt.artifact.resolver.generic.utils.GenericUtils Maven / Gradle / Ivy
The newest version!
package org.metaeffekt.artifact.resolver.generic.utils;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.exception.ExceptionUtils;
import org.apache.http.HttpResponse;
import org.metaeffekt.artifact.resolver.download.WebAccess;
import java.io.File;
import java.io.IOException;
import java.time.Instant;
import java.time.temporal.ChronoUnit;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Supplier;
import static org.metaeffekt.artifact.resolver.generic.utils.MarkerUtils.queryMarker;
@Slf4j
public class GenericUtils {
public static boolean isModifiedInLast24Hours(File file) {
final Instant lastModified = Instant.ofEpochMilli(file.lastModified());
return lastModified.plus(1, ChronoUnit.DAYS).isAfter(Instant.now());
}
public static Consumer getHttpResponseErrorConsumer_invalidateMarker(@NonNull File markerFile, String ref) {
return httpResponse -> {
log.trace("Got http response [{}] while resolving for ref [{}].", httpResponse, ref);
MarkerUtils.invalidateMarkerFor(markerFile, ref);
};
}
/**
* Downloads a file to destination from a given url using the given webaccess. Does NOT handle markers.
*
* @param webAccess webaccess to use for download
* @param url url to download the file from
* @param destinationFile destination file
* @param reference to which overarching artifact is being resolved right now; used for better logging
* @return a file that may contain data or null
*/
public static File downloadFile(@NonNull WebAccess webAccess,
@NonNull String url,
@NonNull File destinationFile,
@NonNull String reference) {
try (WebAccess.WebSession session = webAccess.createSession()) {
log.info("Downloading [{}] for [{}].", url, reference);
Optional downloadedFile = session.downloadFile(
url,
destinationFile,
(httpResponse) -> log.info("Download failed for url [{}] to dest [{}] with [{}].",
url, destinationFile, httpResponse.getStatusLine())
);
return downloadedFile.orElse(null);
} catch (IOException e) {
log.warn("Failed to download url [{}] to dest [{}] for ref [{}] due to exception.",
url, destinationFile.toPath(), reference, e);
return null;
}
}
/**
* Downloads a file to destination from a given url using the given webaccess. This version writes markers.
*
* @param webAccess webAccess to use for download
* @param url url to download the file from
* @param destination destination file
* @param markerFile the marker file corresponding to destination file
* @param reference to which overarching artifact is being resolved right now; used for better logging
* @return a file that may contain data or null
* @deprecated consider using {@link MarkerUtils#attemptDownload(File, String, Supplier)} for better encapsulation
*/
@Deprecated
public static File downloadFile(@NonNull WebAccess webAccess,
@NonNull String url,
@NonNull File destination,
@NonNull File markerFile,
@NonNull String reference) {
// TODO: rewrite / improve: only mark downloads as failed on http failure or exception,
// not when a user aborts the jvm by stopping the program.
// this would be somewhat more involved: should think about which errors to catch.
// get file from marker if possible
final MarkerQueryResult queryResult = queryMarker(markerFile, reference);
if (queryResult.getFoundTarget() != null) {
log.debug("Skipping successful download for ref [{}]: url [{}] to yield [{}]; marker gave [{}].",
reference,
url,
destination.getPath(),
queryResult.getFoundTarget());
return queryResult.getFoundTarget();
} else {
if (queryResult.isAttemptedRecently()) {
// download failed recently. skip.
log.info("Skipping failed download for ref [{}]: url [{}] for destination [{}] failed recently.",
reference,
url,
destination.getPath());
return null;
}
}
// attempt download
MarkerUtils.touchMarker(markerFile, reference);
try (WebAccess.WebSession session = webAccess.createSession()) {
log.info("Downloading [{}] for [{}].", url, reference);
Optional downloadedFile = session.downloadFile(
url,
destination,
getHttpResponseErrorConsumer_invalidateMarker(markerFile, reference)
);
// mark download as success
if (downloadedFile.isPresent()) {
MarkerUtils.markSuccess(downloadedFile.orElse(null), markerFile, reference);
}
return downloadedFile.orElse(null);
} catch (IOException e) {
log.warn("Failed to download [{}] to [{}] for [{}] with [{}].",
url,
destination.getAbsolutePath(),
reference,
ExceptionUtils.getStackTrace(e));
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy