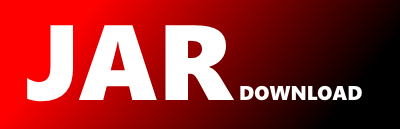
org.metaeffekt.artifact.resolver.maven.MavenArtifactReference Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.maven;
import com.github.packageurl.PackageURL;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import org.apache.commons.lang3.StringUtils;
import org.metaeffekt.artifact.resolver.generic.FileLocation;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.metaeffekt.core.inventory.processor.model.Constants;
@Setter
@Getter
@ToString
public class MavenArtifactReference {
private String artifactId;
private String groupId;
private String version;
private String classifier;
private String type;
private String packaging; // FIXME: not fully integrated and differentiated from type yet
private String observedFileName;
private String observedSha256;
public MavenArtifactReference() {}
public MavenArtifactReference(Artifact artifact) {
// FIXME-CORE: clean this up in core; propose to return value and cache internally
artifact.deriveArtifactId();
artifactId = artifact.getArtifactId();
groupId = artifact.getGroupId();
version = artifact.getVersion();
classifier = artifact.getClassifier();
type = artifact.getType();
// in case the type cannot be determined the default type 'jar' is used
// FIXME-BKL: replace literals with best fitting constants
final String ecosystem = artifact.get("Ecosystem");
if ("maven".equalsIgnoreCase(ecosystem)) {
if (StringUtils.isEmpty(type)) {
type = "jar";
}
}
observedFileName = artifact.getId();
observedSha256 = artifact.get(Constants.KEY_HASH_SHA256);
// in some systems the artifactId is a composition following the pattern
// .-[-classifier].
// modulate artifactId (remove '.' prefix)
if (StringUtils.isNotEmpty(groupId)) {
final String prefix = groupId + ".";
if (artifactId.startsWith(prefix)) {
artifactId = artifactId.substring(prefix.length());
}
}
}
public MavenArtifactReference(PackageURL packageURL) {
if (packageURL.getType().equalsIgnoreCase(PackageURL.StandardTypes.MAVEN)) {
artifactId = packageURL.getName();
groupId = packageURL.getNamespace();
version = packageURL.getVersion();
if (packageURL.getQualifiers() != null) {
classifier = packageURL.getQualifiers().get("classifier");
type = packageURL.getQualifiers().get("type");
} else {
classifier = null;
type = null;
}
} else {
artifactId = null;
groupId = null;
version = null;
classifier = null;
type = null;
}
}
/**
* Copy artifactReference while optionally overriding classifier and packaging.
* @param artifactReference reference to copy most fields from
* @param classifier modify classifier to this value if non-null
* @param packaging modify packaging (and type) to this value if non-null
* @see #modulateAttribute(String, String)
*/
public MavenArtifactReference(MavenArtifactReference artifactReference, String classifier, String packaging) {
this(artifactReference);
this.type = modulateAttribute(artifactReference.type, packaging);
this.classifier = modulateAttribute(artifactReference.classifier, classifier);
}
public MavenArtifactReference(MavenArtifactReference artifactReference) {
this.artifactId = artifactReference.artifactId;
this.groupId = artifactReference.groupId;
this.version = artifactReference.version;
this.classifier = artifactReference.classifier;
this.type = artifactReference.type;
this.packaging = artifactReference.packaging;
this.observedFileName = artifactReference.observedFileName;
this.observedSha256 = artifactReference.observedSha256;
}
/**
* Modules the attribute. Differentiates null (do not override), "" (do overwrite with null), string (overwrite with string).
*
* @param current The current value (not modulated).
* @param modified The override value.
*
* @return The adjusted value.
*/
private String modulateAttribute(String current, String modified) {
if (modified != null) {
if (modified.isEmpty()) {
return null;
}
return modified;
}
return current;
}
public boolean isValid() {
return StringUtils.isNotEmpty(artifactId) && StringUtils.isNotEmpty(groupId) && StringUtils.isNotEmpty(version) &&
!Constants.ASTERISK.equals(version);
}
public String deriveMavenDirectory() {
// maven layout
StringBuilder mavenDirectory = new StringBuilder(getGroupId().replace(".", "/"));
mavenDirectory.append("/").append(getArtifactId());
mavenDirectory.append("/").append(getVersion());
return mavenDirectory.toString();
}
public String deriveFileName() {
StringBuilder name = new StringBuilder(getArtifactId());
name.append("-").append(getVersion());
if (getClassifier() != null) {
name.append("-").append(getClassifier());
}
name.append(".").append(deriveType());
// NOTE: rxbluetooth had quotes in the artifact id
return name.toString().replaceAll("\"", "");
}
private String deriveType() {
if (type == null) return "jar";
return type;
}
public FileLocation deriveFileLocation(String ecosystem) {
FileLocation fileLocation = new FileLocation();
fileLocation.setEcosystem(ecosystem);
fileLocation.setFilename(deriveFileName());
fileLocation.setDispatchName(getArtifactId());
fileLocation.setResourceQualifier(deriveMavenDirectory().replace('/', '.'));
if (!fileLocation.isValid()) {
throw new IllegalStateException("File location invalid: " + fileLocation);
}
return fileLocation;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy