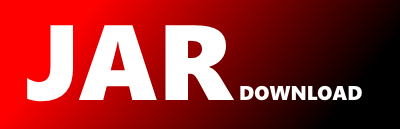
org.metaeffekt.artifact.resolver.maven.MavenPomAdapter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.maven;
import com.github.packageurl.MalformedPackageURLException;
import com.github.packageurl.PackageURL;
import org.apache.commons.lang3.StringUtils;
import org.apache.maven.model.Model;
import org.apache.maven.model.Organization;
import org.apache.maven.model.Parent;
import org.apache.maven.model.io.xpp3.MavenXpp3Reader;
import org.codehaus.plexus.util.xml.pull.XmlPullParserException;
import org.jdom2.JDOMException;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.TreeMap;
public class MavenPomAdapter {
private static final Logger LOG = LoggerFactory.getLogger(MavenPomAdapter.class);
public boolean augmentLicenseMetaData(Artifact artifactToEnrich, MavenArtifactReference mavenArtifactReference, File pomFile) throws IOException, JDOMException {
try (InputStream in = new FileInputStream(pomFile)) {
final Model model = new MavenXpp3Reader().read(in);
// FIXME: add support for extacting package specified licenses.
/*
final List licenses = model.getLicenses();
if (licenses != null && !licenses.isEmpty()) {
for (License license : licenses) {
LicenseMetaData licenseMetaData = new LicenseMetaData();
String licenseName = license.getName();
if (org.springframework.util.StringUtils.hasText(licenseName)) {
licenseMetaData.setLicenseName(licenseName);
licenseMetaData.setLicenseUrl(license.getUrl());
licenseMetaData.setComments(license.getComments());
artifactReference.createArtifactMetaData();
artifactReference.getArtifactMetaData().addLicenseMetaData(licenseMetaData);
}
}
}
*/
String groupId = model.getGroupId();
String version = model.getVersion();
String artifactId = model.getArtifactId();
String packaging = model.getPackaging();
final String name = model.getName();
final String description = model.getDescription();
if (version == null || groupId == null) {
MavenArtifactReference parentRef = extractParentPom(model);
if (parentRef != null) {
if (version == null) {
version = parentRef.getVersion();
}
if (groupId == null) {
groupId = parentRef.getGroupId();
}
}
}
artifactToEnrich.setGroupId(groupId);
artifactToEnrich.setVersion(version);
artifactToEnrich.setComponent(name);
// overwrite with information from reference
if (StringUtils.isNotBlank(mavenArtifactReference.getType())) {
packaging = mavenArtifactReference.getType();
}
// use classifier information from reference
String classifier = mavenArtifactReference.getClassifier();
if (StringUtils.isNotBlank(packaging)) {
if (StringUtils.isNotBlank(classifier)) {
artifactToEnrich.setId(artifactId + "-" + version + "-" + classifier + "." + packaging);
} else {
artifactToEnrich.setId(artifactId + "-" + version + "." + packaging);
}
}
artifactToEnrich.set("Description", description);
final String url = model.getUrl();
if (StringUtils.isEmpty(artifactToEnrich.getUrl())) {
artifactToEnrich.setUrl(url);
}
final Organization organization = model.getOrganization();
final String organizationKey = "Organization";
if (StringUtils.isEmpty(artifactToEnrich.get(organizationKey))) {
if (organization != null && StringUtils.isNotEmpty(organization.getUrl())) {
artifactToEnrich.setUrl(organization.getUrl());
}
if (organization != null && StringUtils.isNotEmpty(organization.getName())) {
artifactToEnrich.setUrl(organization.getName());
}
}
return true;
} catch (Exception e) {
LOG.warn("Could not evaluate POM: " + e.getMessage());
return false;
}
}
public MavenArtifactReference extractParentPom(File pomFile) throws IOException {
if (!pomFile.exists()) return null;
try (final InputStream in = new FileInputStream(pomFile)) {
final Model model = new MavenXpp3Reader().read(in);
return extractParentPom(model);
} catch (XmlPullParserException e) {
throw new IOException(e.getMessage(), e);
}
}
private static MavenArtifactReference extractParentPom(Model model) {
final Parent parent = model.getParent();
if (parent != null) {
try {
final TreeMap treeMap = new TreeMap<>();
treeMap.put("packaging", "pom");
return new MavenArtifactReference(
new PackageURL("maven", parent.getGroupId(), parent.getArtifactId(),
parent.getVersion(), treeMap, null));
} catch (MalformedPackageURLException e) {
throw new RuntimeException(e);
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy