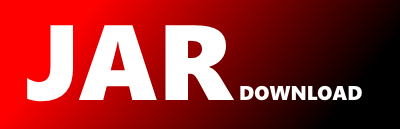
org.metaeffekt.artifact.resolver.maven.MavenRepositoryAdapter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.maven;
import lombok.extern.slf4j.Slf4j;
import org.metaeffekt.artifact.resolver.ResolverResult;
import org.metaeffekt.artifact.resolver.download.WebAccess;
import org.metaeffekt.artifact.resolver.generic.AbstractDownloadingAdapter;
import org.metaeffekt.artifact.resolver.generic.FileLocation;
import org.metaeffekt.artifact.resolver.model.DownloadLocation;
import java.io.File;
import java.io.IOException;
import java.io.UncheckedIOException;
import java.util.Optional;
@Slf4j
public class MavenRepositoryAdapter extends AbstractDownloadingAdapter {
private MavenArtifactResolverConfig resolverConfig;
public MavenRepositoryAdapter(DownloadLocation downloadLocation, WebAccess webAccess, MavenArtifactResolverConfig resolverConfig) {
super(downloadLocation, webAccess);
this.resolverConfig = resolverConfig;
}
public ResolverResult resolvePom(MavenArtifactReference mavenArtifactReference) {
return resolvePart(mavenArtifactReference, "", "pom");
}
public ResolverResult resolveBinaryArtifact(MavenArtifactReference mavenArtifactReference) {
return resolvePart(mavenArtifactReference, null, null);
}
public ResolverResult resolveSourceArtifact(MavenArtifactReference mavenArtifactReference) {
return resolvePart(mavenArtifactReference, "sources", "jar");
}
private ResolverResult resolvePart(MavenArtifactReference artifactReference, String classifierModifier, String packagingModifier) {
final MavenArtifactReference modulatedReference = new MavenArtifactReference(artifactReference, classifierModifier, packagingModifier);
final File file = downloadArtifact(modulatedReference);
if (file != null && file.exists()) {
return resolve(file, null);
}
return null;
}
public File downloadArtifact(MavenArtifactReference artifactRef) {
if (resolverConfig != null && resolverConfig.getRepositoryBaseUrls() != null) {
for (String repositoryBaseUrl : resolverConfig.getRepositoryBaseUrls()) {
try {
File download = download(artifactRef, repositoryBaseUrl);
if (download != null) {
return download;
}
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
}
return null;
}
private File download(MavenArtifactReference artifactRef, String repositoryBaseUrl) throws IOException {
// FIXME: there are issues with handling "NA" classifiers in ref. might relate to deriveFileName.
final String mavenDirectory = artifactRef.deriveMavenDirectory();
final String filename = artifactRef.deriveFileName();
final FileLocation fileLocation = artifactRef.deriveFileLocation("maven");
final File file = deriveDownloadFile(fileLocation);
final File markerFile = deriveMarkerFile(fileLocation, repositoryBaseUrl);
final String uri = repositoryBaseUrl + mavenDirectory + "/" + filename;
if (!file.exists()) {
// compute relative path to construct marker file path
// do not reattempt download as long as a recent (less than a day old) marker file exists
if (hasRecentFailedDownloadAttempt(markerFile)) {
log.info("Skipping failed download of [{}]. Recent attempt failed for file [{}].",
uri, file.getPath());
return null;
}
removeMarker(markerFile);
try (WebAccess.WebSession session = getWebAccess().createSession()) {
log.info("Downloading: [{}]", uri);
Optional downloadFile = session.downloadFile(uri, file, manageMarkerErrorResponseConsumer(markerFile));
// TODO: check hashes against a downloaded md5 and or sha1 file for better integrity
return downloadFile.orElse(null);
}
} else {
// TODO: "file already exists" is dangerous logic in case of interrupted downloads.
// This could be improved with some sanity checks.
// (for other resolvers as well)
log.debug("Skipping successful download of [{}] for existing file [{}].", uri, file.getPath());
}
return file;
}
/*
public File downloadPomHierarchy(File aggregatorDir, ArtifactReference gavReference, File downloadDir, File analysisDir) {
try {
MavenPomAdapter mavenPomAdapter = new MavenPomAdapter();
// construct a artifact reference that points to the corresponding POM
ArtifactReference pomReference = new ArtifactReference(gavReference);
pomReference.setClassifier(null);
pomReference.setPackaging("pom");
// download POM and unpack; recurse
File artifactPomFile = downloadArtifact(pomReference, downloadDir);
if (artifactPomFile != null && artifactPomFile.exists()) {
// create analysis folder
ArchiveReference pomArchiveReference = pomReference.deriveArchiveReference(downloadDir, analysisDir);
pomArchiveReference.unpackArchiveOrCopyFile();
if (aggregatorDir != null) {
org.metaeffekt.core.util.FileUtils.copyDirectory(
pomArchiveReference.getUnpackedDir(), aggregatorDir);
} else {
aggregatorDir = pomArchiveReference.getUnpackedDir();
}
}
File file = artifactPomFile;
while (file != null) {
ArtifactReference parentPomReference = mavenPomAdapter.extractParentPom(file);
file = null;
if (parentPomReference != null) {
file = downloadPomHierarchy(aggregatorDir, parentPomReference, downloadDir, analysisDir);
}
}
return artifactPomFile;
} catch (Exception e) {
log.error("Cannot download POM for {}.", gavReference, e);
}
return null;
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy