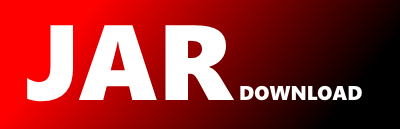
org.metaeffekt.artifact.resolver.maven.attic.MavenCentralAdapter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.maven.attic;
import org.apache.commons.io.IOUtils;
import org.json.JSONArray;
import org.json.JSONObject;
import org.metaeffekt.artifact.resolver.download.WebAccess;
import org.metaeffekt.artifact.resolver.generic.AbstractDownloadingAdapter;
import org.metaeffekt.artifact.resolver.generic.FileLocation;
import org.metaeffekt.artifact.resolver.maven.MavenArtifactReference;
import org.metaeffekt.artifact.resolver.model.DownloadLocation;
import org.metaeffekt.core.util.FileUtils;
import org.springframework.util.Base64Utils;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringWriter;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
public class MavenCentralAdapter extends AbstractDownloadingAdapter {
public MavenCentralAdapter(DownloadLocation downloadLocation, WebAccess webAccess) {
super(downloadLocation, webAccess);
}
private static final Map EC_CLASSIFIER_MAP = new HashMap<>();
static {
EC_CLASSIFIER_MAP.put(".pom", null);
EC_CLASSIFIER_MAP.put(".jar", null);
EC_CLASSIFIER_MAP.put("-sources.jar", "sources");
EC_CLASSIFIER_MAP.put("-javadoc.jar", "javadoc");
EC_CLASSIFIER_MAP.put(".zip", null);
EC_CLASSIFIER_MAP.put(".tar.gz", null);
EC_CLASSIFIER_MAP.put(".pdf", null);
EC_CLASSIFIER_MAP.put("-doc.jar", "doc");
EC_CLASSIFIER_MAP.put("-test.jar", "test");
EC_CLASSIFIER_MAP.put("-tests.jar", "tests");
}
private static final Map EC_PACKAGING_MAP = new HashMap<>();
static {
EC_PACKAGING_MAP.put(".pom", "pom");
EC_PACKAGING_MAP.put(".jar", "jar");
EC_PACKAGING_MAP.put("-sources.jar", "jar");
EC_PACKAGING_MAP.put("-javadoc.jar", "jar");
EC_PACKAGING_MAP.put(".zip", "zip");
EC_PACKAGING_MAP.put(".tar.gz", "tar.gz");
EC_PACKAGING_MAP.put(".pdf", "pdf");
EC_PACKAGING_MAP.put("-doc.jar", "jar");
EC_PACKAGING_MAP.put("-test.jar", "jar");
EC_PACKAGING_MAP.put("-tests.jar", "jar");
}
public Set queryArtifacts(MavenArtifactReference artifactReference) throws IOException {
final String serviceUrl = "https://search.maven.org/solrsearch/select";
final StringBuilder query = new StringBuilder("q=");
boolean andRequired = false;
if (artifactReference.getGroupId() != null) {
if (andRequired) {
query.append("+AND+");
}
query.append(replaceVar("g%3A%22${var}%22", artifactReference.getGroupId()));
andRequired = true;
}
if (artifactReference.getArtifactId() != null) {
if (andRequired) {
query.append("+AND+");
}
query.append(replaceVar("a%3A%22${var}%22", artifactReference.getArtifactId()));
andRequired = true;
}
/*
if (artifactReference.getVersion() != null) {
if (andRequired) {
query.append("+AND+");
}
query.append(replaceVar("v%3A%22${var}%22", artifactReference.getVersion()));
}
*/
final String uri = serviceUrl + "?" + query + "&core=gav&rows=20&wt=json";
final String encodedQuery = Base64Utils.encodeToString(query.toString().getBytes());
FileLocation fileLocation = new FileLocation();
fileLocation.setEcosystem("solrsearch");
fileLocation.setFilename(encodedQuery);
fileLocation.setResourceQualifier(artifactReference.deriveFileName());
fileLocation.setDispatchName(artifactReference.deriveFileName());
final File file = deriveDownloadFile(fileLocation);
final File markerFile = deriveMarkerFile(fileLocation, serviceUrl);
getWebAccess().createSession().downloadFile(uri, file, manageMarkerErrorResponseConsumer(markerFile));
if (file.exists()) {
return parse(FileUtils.readFileToString(file, FileUtils.ENCODING_UTF_8));
}
return null;
}
public Set parse(String json) {
JSONObject object = new JSONObject(json);
JSONObject response = object.getJSONObject("response");
JSONArray docs = response.getJSONArray("docs");
Set set = new HashSet<>();
for (int i = 0; i < docs.length(); i++) {
JSONObject doc = docs.getJSONObject(i);
JSONArray classifiers = doc.getJSONArray("ec");
for (int j = 0; j < classifiers.length(); j++) {
String classifier = EC_CLASSIFIER_MAP.get(classifiers.getString(j));
String packaging = EC_PACKAGING_MAP.get(classifiers.getString(j));
MavenArtifactReference artifactReference = new MavenArtifactReference();
artifactReference.setPackaging(packaging);
artifactReference.setArtifactId(doc.getString("a"));
artifactReference.setGroupId(doc.getString("g"));
artifactReference.setVersion(doc.getString("v"));
artifactReference.setVersion(doc.getString("v"));
artifactReference.setClassifier(classifier);
set.add(artifactReference);
}
}
return set;
}
private String replaceVar(String string, String value) {
return string.replace("${var}", value);
}
public String convert(InputStream in, String encoding, boolean handleStream) throws IOException {
try {
StringWriter writer = new StringWriter();
IOUtils.copy(in, writer, encoding);
return writer.toString();
} finally {
if (handleStream) {
IOUtils.closeQuietly(in);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy