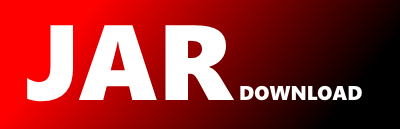
org.metaeffekt.artifact.resolver.model.ArtifactPartResolver Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.model;
import lombok.Getter;
import org.apache.commons.lang3.StringUtils;
import org.metaeffekt.artifact.resolver.ResolverResult;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import java.util.function.Function;
import java.util.function.Supplier;
public class ArtifactPartResolver {
@Getter
private final Artifact artifact;
@Getter
private final ArtifactPartType artifactPartType;
private final Supplier artifactPartSupplier;
private final Function enrichmentFunction;
public ArtifactPartResolver(Artifact artifact,
ArtifactPartType artifactPartType,
Supplier artifactSupplier,
Function enrichmentFunction) {
this.artifact = artifact;
this.artifactPartType = artifactPartType;
this.artifactPartSupplier = artifactSupplier;
this.enrichmentFunction = enrichmentFunction;
}
public ResolvedArtifactPart get() {
ResolverResult resolverResult;
try {
resolverResult = artifactPartSupplier.get();
// in case the supplier says null, we respect this. Nothing to resolve
if (resolverResult == null) return null;
} catch (Exception e) {
// FIXME: revise this level of exception handling
// in case of an unexpected error we take note; chekc whether the exception should be logged.
resolverResult = new ResolverResult(null, "Cannot resolve.");
}
// create ResolvedArtifactPart based on resolver results and member variables
final ResolvedArtifactPart resolvedArtifactPart =
new ResolvedArtifactPart(resolverResult, artifact, artifactPartType);
// apply resolver-specific enrichment
resolvedArtifactPart.applyEnrichment(enrichmentFunction);
final Artifact enrichedArtifact = resolvedArtifactPart.getEnrichedArtifact();
// manage error supplied on supplier/resolver-level
final String error = resolverResult.getErrorMessage();
if (StringUtils.isNotBlank(error)) {
String errorAttribute = artifactPartType.modulateAttributeInContext("Errors");
enrichedArtifact.append(errorAttribute, error, ", ");
}
return resolvedArtifactPart;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy