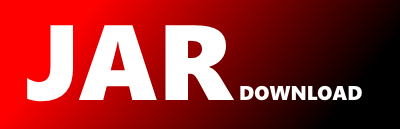
org.metaeffekt.artifact.resolver.npm.NpmRepositoryAdapter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.npm;
import org.metaeffekt.artifact.resolver.ResolverResult;
import org.metaeffekt.artifact.resolver.download.WebAccess;
import org.metaeffekt.artifact.resolver.generic.AbstractDownloadingAdapter;
import org.metaeffekt.artifact.resolver.generic.FileLocation;
import org.metaeffekt.artifact.resolver.model.DownloadLocation;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.IOException;
import java.util.Optional;
public class NpmRepositoryAdapter extends AbstractDownloadingAdapter {
private static final Logger LOG = LoggerFactory.getLogger(NpmRepositoryAdapter.class);
public NpmRepositoryAdapter(DownloadLocation downloadLocation, WebAccess webAccess) {
super(downloadLocation, webAccess);
}
private File downloadArtifact(NpmArtifactReference reference, String urlPart) {
try {
// FIXME: move into config; support multiple repos
final String repositoryUrl = "https://registry.npmjs.org/";
File download = download(reference, repositoryUrl, urlPart);
if (download != null) {
return download;
}
} catch (Exception e) {
// ignore; missing pom will be handled by caller
}
return null;
}
private File download(NpmArtifactReference reference, String repositoryUrl, String urlPath) throws IOException {
final String uri = repositoryUrl + urlPath;
final FileLocation fileLocation = reference.deriveFileLocation();
final File file = deriveDownloadFile(fileLocation);
final File markerFile = deriveMarkerFile(fileLocation, repositoryUrl);
if (!file.exists()) {
if (uri.startsWith("http")) {
// do not reattempt download as long as a recent (less than a day old) marker file exists
if (hasRecentFailedDownloadAttempt(markerFile)) {
LOG.info("Skipping download [{}]. Recent attempt failed: [{}]", uri, file.getAbsolutePath());
return null;
}
// manage markers; remove complete folder once we decided to attempt a new download
removeMarker(markerFile);
try (WebAccess.WebSession session = getWebAccess().createSession()) {
LOG.info("Downloading: [{}]", uri);
Optional downloadFile = session.downloadFile(uri, file, manageMarkerErrorResponseConsumer(markerFile));
return downloadFile.orElse(null);
}
}
} else {
LOG.debug("Skipping download [{}]. File already exists: [{}]", uri, file.getAbsolutePath());
}
return file;
}
public ResolverResult resolveSourceArchive(NpmArtifactReference artifactReference) {
final File file = downloadArtifact(artifactReference, artifactReference.deriveUrlPart());
return resolve(file, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy