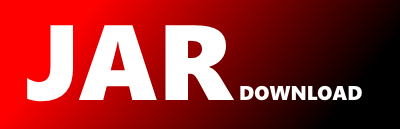
org.metaeffekt.artifact.resolver.rpm.index.RpmIndexPackageData Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.rpm.index;
import lombok.Getter;
import lombok.ToString;
import java.util.HashSet;
import java.util.Objects;
import java.util.Set;
@ToString
public class RpmIndexPackageData {
@Getter
private String name;
@Getter
private String version;
@Getter
private String release;
@Getter
private String archOrSource;
@Getter
private String suffix;
public RpmIndexPackageData(String url) {
String filename = url.substring(url.lastIndexOf("/") + 1);
int suffixDotIndex = filename.lastIndexOf(".");
suffix = filename.substring(suffixDotIndex + 1);
String remainder = filename.substring(0, suffixDotIndex);
int archDotIndex = remainder.lastIndexOf(".");
archOrSource = remainder.substring(archDotIndex + 1);
validateArchOrSource(archOrSource);
remainder = remainder.substring(0, archDotIndex);
int releaseDashIndex = remainder.lastIndexOf("-");
release = remainder.substring(releaseDashIndex + 1);
remainder = remainder.substring(0, releaseDashIndex);
int versionDashIndex = remainder.lastIndexOf("-");
version = remainder.substring(versionDashIndex + 1);
name = remainder.substring(0, versionDashIndex);
}
public static Set filter(Set packageSet, String versionAndRelease, String archOrSource) {
final Set filteredSet = new HashSet<>();
for (RpmIndexPackageData rpmIndexPackageData : packageSet) {
// FIXME: can we improve this; currently we produce wrong combinations to match the input data
final String candidateVersionAndRelease = rpmIndexPackageData.getVersion() + "-" + rpmIndexPackageData.getRelease();
final String candidateVersionAndReleaseArchOrSource = candidateVersionAndRelease + "." + rpmIndexPackageData.getArchOrSource();
if (candidateVersionAndRelease.equals(versionAndRelease) || candidateVersionAndReleaseArchOrSource.equals(versionAndRelease)) {
if (archOrSource == null || archOrSource.equals(rpmIndexPackageData.getArchOrSource())) {
filteredSet.add(rpmIndexPackageData);
}
}
}
// FIXME: we should consider to pull similar sources as a fallback; required for project elaborating on licenses.
boolean enableFallback = false;
if (enableFallback) {
// ugly fallback / does not match full version; index too sparse
if (filteredSet.isEmpty()) {
for (RpmIndexPackageData rpmIndexPackageData : packageSet) {
final String candidateVersion = rpmIndexPackageData.getVersion();
if (versionAndRelease.startsWith(candidateVersion + "-")) {
if (archOrSource == null || archOrSource.equals(rpmIndexPackageData.getArchOrSource())) {
filteredSet.add(rpmIndexPackageData);
break;
}
}
}
}
// ugly fallback / only match name
if (filteredSet.isEmpty()) {
for (RpmIndexPackageData rpmIndexPackageData : packageSet) {
filteredSet.add(rpmIndexPackageData);
break;
}
}
}
return filteredSet;
}
private void validateArchOrSource(String archOrSource) {
if (!archOrSource.equals("src") &&
!archOrSource.equals("noarch") &&
!archOrSource.equals("x86_64") &&
!archOrSource.equals("i686") &&
!archOrSource.equals("aarch64") &&
!archOrSource.equals("ppc64le")) {
throw new IllegalStateException(archOrSource);
}
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
final RpmIndexPackageData that = (RpmIndexPackageData) o;
return that.toFilename().equals(this.toFilename());
}
public String toFilename() {
return name + "-" + version + "-" + release + "." + archOrSource + "." + suffix;
}
@Override
public int hashCode() {
return Objects.hash(toFilename());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy