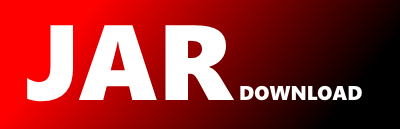
org.metaeffekt.artifact.resolver.deb.ControlFileSourceReference Maven / Gradle / Ivy
package org.metaeffekt.artifact.resolver.deb;
import lombok.Getter;
import lombok.NonNull;
import java.util.*;
public class ControlFileSourceReference {
/**
* Raw filename as spliced from the control file.
*/
@Getter
private final String name;
/**
* Size as spliced from a line of the control file.
*
* -1 if there was a parsing error; don't really want to crash because of this.
* I'd rather handle checksum mismatches lateron.
*/
@Getter
private final int size;
/**
* Contains pairs of checksum type and the provided checksum that came with it.
*
* Note that the type string is toLowerCase'd, cut from the end of the checksums field name in the control file.
* This is because fieldnames in debian control files are to be considered case-insensitive.
* Significant such types include:
*
* - sha256
* - sha1
* - md5 (substituted when reading from {@code .dcs}'s {@code Files} field
*
*/
private final Map checksumTypeToChecksum = new HashMap<>();
public ControlFileSourceReference(@NonNull String name,
int size) {
this.name = name;
this.size = size;
}
/**
* Returns registered types of checksum for this source reference.
*
* Useful if unsure which one to use (for example fallbacks).
* @return a collection of known checksum types
*/
public Collection getChecksumTypes() {
return Collections.unmodifiableCollection(checksumTypeToChecksum.keySet());
}
/**
* Get the checksum value for a particular type.
* @param type type of checksum to retrieve, where the string is the ending of the control's Checksums field or md5
* see {@link #checksumTypeToChecksum}
* @return returns the value of the checksum or null if no value is found.
*/
public String getChecksum(String type) {
return checksumTypeToChecksum.get(type);
}
/**
* Adds a checksum to storage. Little checkins is performed in the method.
* @param type type of checksum, see {@link #checksumTypeToChecksum}
* @param checksum checksum value (as read from control file, should be hex)
* @return the previously associated value as in {@link Map#put(Object, Object)}
*/
public String addChecksum(@NonNull String type, String checksum) {
if (checksum == null) {
// useful to handle this special case to keep the map clean for things like listing registered types
return checksumTypeToChecksum.remove(type.toLowerCase(Locale.ENGLISH));
}
return checksumTypeToChecksum.put(type.toLowerCase(Locale.ENGLISH), checksum);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy