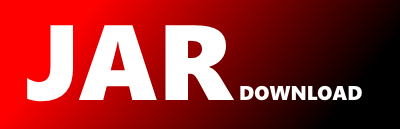
org.metaeffekt.artifact.resolver.manager.execenv.EnvironmentManager Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.manager.execenv;
import io.fabric8.kubernetes.client.Config;
import io.fabric8.kubernetes.client.KubernetesClient;
import io.fabric8.kubernetes.client.KubernetesClientBuilder;
import io.fabric8.kubernetes.client.VersionInfo;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
import org.metaeffekt.artifact.resolver.manager.execenv.exception.EnvironmentInitializationFailure;
import org.metaeffekt.core.container.control.kubernetesapi.NamespaceHandle;
import java.lang.reflect.InvocationTargetException;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.concurrent.atomic.AtomicBoolean;
/**
* An overarching controller for keeping environments alive for the liftime of the curent jvm.
*
* The point of this is to keep track of open environments, not opening hundreds and exhausting resources.
*
* These can then be used by artifact resolvers.
*/
@Slf4j
public class EnvironmentManager {
/**
* This will act as an LRU cache.
*/
private final LinkedHashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy