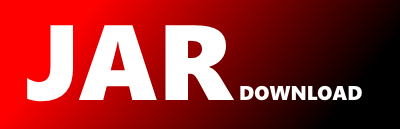
org.metaeffekt.artifact.resolver.url.UrlArtifactResolver Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.artifact.resolver.url;
import lombok.NonNull;
import org.apache.commons.lang3.StringUtils;
import org.metaeffekt.artifact.resolver.download.WebAccess;
import org.metaeffekt.artifact.resolver.generic.AbstractArtifactResolver;
import org.metaeffekt.artifact.resolver.model.*;
import org.metaeffekt.core.inventory.processor.model.Artifact;
import java.io.File;
import java.util.Collection;
import java.util.HashSet;
import static org.metaeffekt.artifact.resolver.model.ArtifactPartType.*;
public class UrlArtifactResolver extends AbstractArtifactResolver {
private final UrlDownloadAdapter urlDownloadAdapter;
public UrlArtifactResolver(DownloadLocation downloadLocation, WebAccess webAccess) {
this.urlDownloadAdapter = new UrlDownloadAdapter(downloadLocation, webAccess);
}
@Override
public ArtifactPartResolvers collectResolvers(@NonNull Artifact artifact) {
final Collection parts = new HashSet<>();
addUrlResources(parts, artifact);
return new ArtifactPartResolvers(parts);
}
private void addUrlResources(Collection parts, Artifact artifact) {
final String binaryArtifactUrl = artifact.get("Binary Artifact - URL");
final String sourceArtifactUrl = artifact.get("Source Artifact - URL");
final String sourceArchiveUrl = artifact.get("Source Archive - URL");
final String descriptorUrl = artifact.get("Descriptor - URL");
addPartResolvers(parts, artifact, BINARY_ARTIFACT, binaryArtifactUrl);
addPartResolvers(parts, artifact, SOURCE_ARTIFACT, sourceArtifactUrl);
addPartResolvers(parts, artifact, SOURCE_ARCHIVE, sourceArchiveUrl);
addPartResolvers(parts, artifact, DESCRIPTOR, descriptorUrl);
}
private void addPartResolvers(Collection parts, Artifact artifact, ArtifactPartType artifactPartType, String partUrl) {
if (StringUtils.isNotEmpty(partUrl)) {
final UrlArtifactReference urlArtifactReference = new UrlArtifactReference(partUrl);
parts.add(new ArtifactPartResolver(artifact, artifactPartType,
// specific supplier
() -> urlDownloadAdapter.resolve(urlArtifactReference),
// specific enrichment function
this::enrichArtifact
)
);
}
}
private Artifact enrichArtifact(ResolvedArtifactPart arp) {
final Artifact enrichedArtifact = new Artifact(arp.getOriginalArtifact());
// in case a file was resolved, add an enriched artifact
final File resolvedFile = arp.getResolvedFile();
if (resolvedFile != null) {
final String absolutePath = resolvedFile.getAbsolutePath();
// modulate attribute before transfer to convey part-content
final String partAttribute = arp.getArtifactPartType().modulateAttributeInContext("Path");
// transfer path attribute
enrichedArtifact.set(partAttribute, absolutePath);
}
return enrichedArtifact;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy