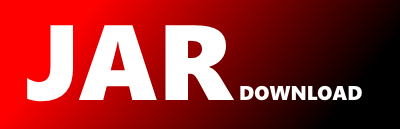
org.metaeffekt.common.notice.model.NoticeParameters Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metaeffekt.common.notice.model;
import org.metaeffekt.common.notice.utils.NoticeUtils;
import org.yaml.snakeyaml.DumperOptions;
import org.yaml.snakeyaml.Yaml;
import org.yaml.snakeyaml.introspector.Property;
import org.yaml.snakeyaml.nodes.*;
import org.yaml.snakeyaml.representer.Representer;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.*;
import java.util.stream.Collectors;
public class NoticeParameters {
private static final String NOTICE_PARAMETERS_REPRESENTATION = "!!" + NoticeParameters.class.getName();
private static final String COMPONENT_DEFINITION_REPRESENTATION = "!!" + ComponentDefinition.class.getName();
/**
* Simple representer to order name attribute to top. Additionally, the representer omits empty values.
*/
public static final Representer YAML_REPRESENTER = new Representer(new DumperOptions()) {
public static final String ATTRIBUTE_NAME = "name";
public static final String ATTRIBUTE_COPYRIGHTS = "copyrights";
protected MappingNode representJavaBean(Set properties, Object javaBean) {
final MappingNode mappingNode = super.representJavaBean(properties, javaBean);
NodeTuple nameNodeTuple = null;
NodeTuple copyrightNodeTuple = null;
final Set disposableNodes = new HashSet<>();
for (NodeTuple nodeTuple : mappingNode.getValue()) {
final Node keyNode = nodeTuple.getKeyNode();
if (keyNode instanceof ScalarNode) {
final ScalarNode sn = (ScalarNode) keyNode;
if (ATTRIBUTE_NAME.equals(sn.getValue())) {
nameNodeTuple = nodeTuple;
}
final Node valueNode = nodeTuple.getValueNode();
if (valueNode instanceof ScalarNode) {
final ScalarNode svn = (ScalarNode) valueNode;
if (svn.getValue() == null || svn.getValue().equals("null")) {
disposableNodes.add(nodeTuple);
} else {
if (svn.getValue().equals("false")) {
disposableNodes.add(nodeTuple);
}
}
}
if (valueNode instanceof SequenceNode) {
final SequenceNode svn = (SequenceNode) valueNode;
if (svn.getValue() == null || svn.getValue().isEmpty()) {
disposableNodes.add(nodeTuple);
}
if (ATTRIBUTE_COPYRIGHTS.equals(sn.getValue())) {
copyrightNodeTuple = nodeTuple;
}
}
}
}
if (nameNodeTuple != null) {
mappingNode.getValue().remove(nameNodeTuple);
mappingNode.getValue().add(0, nameNodeTuple);
}
if (copyrightNodeTuple != null) {
mappingNode.getValue().remove(copyrightNodeTuple);
mappingNode.getValue().add(copyrightNodeTuple);
}
mappingNode.getValue().removeAll(disposableNodes);
return mappingNode;
}
};
/**
* The uris serves as identified for the notice parameter
*/
private String uri;
/**
* Top-level {@link ComponentDefinition}.
*/
private ComponentDefinition component;
/**
* List of {@link ComponentDefinition}s for the subcomponents.
*/
private List subcomponents = Collections.emptyList();
private UnassignedInformation unassignedInformation;
public ComponentDefinition getComponent() {
return component;
}
public ComponentDefinition findComponent(ComponentDefinition component) {
if (this.component.equals(component)) return this.component;
for (ComponentDefinition subcomponent : this.subcomponents) {
if (subcomponent.equals(component)) {
return subcomponent;
}
}
return null;
}
public List getAllAssociatedLicense() {
List associatedLicenses = new ArrayList<>();
if (component != null) {
associatedLicenses.addAll(component.getAssociatedLicenses());
}
if (subcomponents != null) {
for (ComponentDefinition subcomponent : subcomponents) {
associatedLicenses.addAll(subcomponent.getAssociatedLicenses());
}
}
return associatedLicenses;
}
public List getSubcomponents() {
return subcomponents;
}
public void setComponent(ComponentDefinition components) {
this.component = components;
}
public void setSubcomponents(List subcomponents) {
this.subcomponents = subcomponents;
}
public void setUnassignedInformation(UnassignedInformation unassignedInformation) {
this.unassignedInformation = unassignedInformation;
}
public UnassignedInformation getUnassignedInformation() {
return unassignedInformation;
}
@Override
public String toString() {
return "NoticeParameters{" +
"uri='" + uri + '\'' +
", component=" + component +
", subcomponents=" + subcomponents +
", unassignedInformation=" + unassignedInformation +
'}';
}
public static NoticeParameters readYaml(File file) throws IOException {
try (FileReader reader = new FileReader(file)) {
NoticeParameters param = new Yaml().loadAs(reader, NoticeParameters.class);
return param;
}
}
public static NoticeParameters readYaml(String string) {
return new Yaml().loadAs(string, NoticeParameters.class);
}
public String toYamlString() {
String string = new Yaml(YAML_REPRESENTER).dumpAs(this, null, DumperOptions.FlowStyle.BLOCK).replace(
NOTICE_PARAMETERS_REPRESENTATION, "").trim();
if (string.equals("null")) return null;
if (string.equals("{}")) return null;
if (string.equals("[]")) return null;
return string;
}
public String toYamlComponentString() {
String string = new Yaml(YAML_REPRESENTER).dumpAs(component, null, DumperOptions.FlowStyle.BLOCK).replace(
COMPONENT_DEFINITION_REPRESENTATION, "").trim();
if (string.equals("null")) return null;
if (string.equals("{}")) return null;
if (string.equals("[]")) return null;
return string;
}
public String toYamlSubcomponentsString() {
String string = new Yaml(YAML_REPRESENTER).dumpAs(subcomponents, null, DumperOptions.FlowStyle.BLOCK).replace(
"- " + COMPONENT_DEFINITION_REPRESENTATION + "\n ", "- ").trim();
if (string.equals("null")) return null;
if (string.equals("{}")) return null;
if (string.equals("[]")) return null;
return string;
}
public String getUri() {
return uri;
}
public List aggregateAssociatedLicenses() {
final Set aggregated = new HashSet<>();
if (getComponent() != null) {
aggregated.addAll(getComponent().getAssociatedLicenses());
}
if (getSubcomponents() != null) {
for (ComponentDefinition componentDefinition : getSubcomponents()) {
aggregated.addAll(componentDefinition.getAssociatedLicenses());
}
}
return aggregated.stream().sorted(String::compareToIgnoreCase).collect(Collectors.toList());
}
public List aggregateCopyrights() {
final Set aggregated = new HashSet<>();
if (unassignedInformation != null && unassignedInformation.getCopyrights() != null) {
aggregated.addAll(unassignedInformation.getCopyrights());
}
if (component != null && component.getCopyrights() != null) {
aggregated.addAll(component.getCopyrights());
}
for (ComponentDefinition componentDefinition : getSubcomponents()) {
aggregated.addAll(componentDefinition.getCopyrights());
}
return NoticeUtils.normalizeCopyrights(aggregated);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy