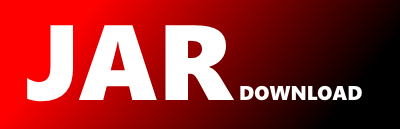
com.metreeca.mark.Opts Maven / Gradle / Ivy
/*
* Copyright © 2019-2023 Metreeca srl
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.metreeca.mark;
import org.apache.maven.plugin.logging.Log;
import org.jetbrains.annotations.Nullable;
import java.beans.IntrospectionException;
import java.beans.Introspector;
import java.lang.reflect.InvocationTargetException;
import java.nio.file.Path;
import java.util.*;
import java.util.function.BiFunction;
import java.util.function.Function;
import static java.util.Arrays.stream;
import static java.util.function.Predicate.not;
/**
* Site generation options.
*/
public interface Opts {
/**
* @return the path of source site folder
*/
public Path source();
/**
* @return the path of target site folder
*/
public Path target();
/**
* @return the source-relative path of the default site layout
*/
public Path layout();
/**
* @return flag indicating whether to generate a README.md file in the project base folder from {@link #source()}
* source}/index.md}
*/
public boolean readme();
/**
* @return the global variables
*/
public Map global();
/**
* @param path the dotted path of a global variable
* @param the expected value type
*
* @return the optional value of the global variable identified by {@code path} or an empty optional is no matching
* value is found
*
* @throws NullPointerException if either {@code type} or {@code path} is null
*/
public default Optional global(final Class type, final String path) {
if ( type == null ) {
throw new NullPointerException("null type");
}
if ( path == null ) {
throw new NullPointerException("null path");
}
final BiFunction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy