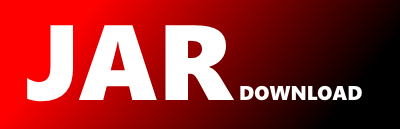
com.microsoft.azure.functions.annotation.HttpTrigger Maven / Gradle / Ivy
Show all versions of azure-functions-java-library Show documentation
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.functions.annotation;
import com.microsoft.azure.functions.HttpMethod;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
*
* The HttpTrigger annotation is applied to Azure functions that will be triggered by a call to the
* HTTP endpoint that the function is located at. The HttpTrigger annotation should be applied to a
* method parameter of one of the following types:
*
*
*
* - {@link com.microsoft.azure.functions.HttpRequestMessage HttpRequestMessage<T>}
* - Any native Java types such as int, String, byte[]
* - Nullable values using Optional<T>
* - Any POJO type
*
*
*
* For example:
*
*
*
* {@literal @}FunctionName("hello")
* public HttpResponseMessage<String> helloFunction(
* {@literal @}HttpTrigger(name = "req",
* methods = {HttpMethod.GET},
* authLevel = AuthorizationLevel.ANONYMOUS) HttpRequestMessage<Optional<String>> request
* ) {
* ....
* }
*
*
*
* In this code snippet you will observe that we have a function annotated with
* {@code @FunctionName("hello")}, which indicates that this function will be available at the
* endpoint /api/hello. The name of the method itself, in this case {@code helloFunction} is
* irrelevant for all intents and purposes related to Azure Functions. Note however that the method
* return type is {@link com.microsoft.azure.functions.HttpResponseMessage}, and that the first
* argument into the function is an {@link com.microsoft.azure.functions.HttpRequestMessage} with
* generic type {@code Optional}. This indicates that the body of the request will
* potentially contain a String value.
*
*
*
* Most important of all however is the {@code @HttpTrigger} annotation that has been applied to
* this argument. In this annotation you'll note that it has been given a name, as well as told what
* type of requests it supports (in this case, only HTTP GET requests), and that the
* {@link AuthorizationLevel} is anonymous, allowing access to anyone who can call the endpoint.
*
*
*
* The {@code HttpTrigger} can be further customised by providing a custom {@link #route()}, which
* allows for custom endpoints to be specified, and for these endpoints to be parameterized with
* arguments being bound to arguments provided to the function at runtime.
*
*
*
* The following example shows a Java function that looks for a name parameter either in the query
* string (HTTP GET) or the body (HTTP POST) of the HTTP request. Notice that the return value is
* used for the output binding, but a return value attribute isn't required.
*
*
*
* {@literal @}FunctionName("readHttpName")
* public String readName(
* {@literal @}HttpTrigger(name = "req",
* methods = {HttpMethod.GET, HttpMethod.POST}, authLevel = AuthorizationLevel.ANONYMOUS)
* final HttpRequestMessage<Optional<String>> request) {
* String name = request.getBody().orElseGet(() -> request.getQueryParameters().get("name"));
* return name == null ?
* "Please pass a name on the query string or in the request body" :
* "Hello " + name;
* }
*
*
* @see com.microsoft.azure.functions.HttpRequestMessage
* @see com.microsoft.azure.functions.HttpResponseMessage
* @since 1.0.0
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.PARAMETER)
public @interface HttpTrigger {
/**
* The variable name used in function code for the request or request body.
*
* @return The variable name used in function code for the request or request body.
*/
String name();
/**
*
* Defines how Functions runtime should treat the parameter value. Possible values are:
*
*
* - "": get the value as a string, and try to deserialize to actual parameter type like
* POJO
* - string: always get the value as a string
* - binary: get the value as a binary data, and try to deserialize to actual parameter type
* byte[]
*
*
* @return The dataType which will be used by the Functions runtime.
*/
String dataType() default "";
/**
*
* Defines the route template, controlling which request URLs your function will respond to. The
* default value if no route is provided is the function name specified in the
* {@link FunctionName} annotation, applied to each Azure Function.
*
*
*
* By default when you create a function for an HTTP trigger, or WebHook, the function is
* addressable with a route of the form
* {@code http://<yourapp>.azurewebsites.net/api/<funcname>}. You can customize this
* route using this route property. For example, a route of
* {@code "products/{category:alpha}/{id:int}"} would mean that the function is now addressable
* with the following route instead of the original route:
* {@code http://<yourapp>.azurewebsites.net/api/products/electronics/357}, which allows the
* function code to support two parameters in the address: category and id. By specifying the
* route in this way, developers can then add the additional route arguments as arguments into the
* function by using the {@link BindingName} annotation. For example:
*
*
*
* {@literal @}FunctionName("routeTest")
* public HttpResponseMessage<String> routeTest(
* {@literal @}HttpTrigger(name = "req",
* methods = {HttpMethod.GET},
* authLevel = AuthorizationLevel.ANONYMOUS,
* route = "products/{category:alpha}/{id:int}")
* HttpRequestMessage<Optional<String>> request,
* {@literal @}BindingName("category") String category,
* {@literal @}BindingName("id") int id,
* final ExecutionContext context
* ) {
* ....
* context.getLogger().info("We have " + category + " with id " + id);
* ....
* }
*
*
*
* For more details on the route syntax, refer to the
* online documentation.
*
*
* @return The route template to use for the annotated function.
*/
String route() default "";
/**
* An array of the HTTP methods to which the function responds. If not specified, the function
* responds to all HTTP methods.
*
* @return An array containing all valid HTTP methods.
*/
HttpMethod[] methods() default {};
/**
*
* Determines what keys, if any, need to be present on the request in order to invoke the
* function. The authorization level can be one of the following values:
*
*
*
* - anonymous: No API key is required.
* - function: A function-specific API key is required. This is the default
* value if none is provided.
* - admin: The master key is required.
*
*
*
* For more information, see the documentation
* about authorization keys.
*
*
* @return An {@link AuthorizationLevel} value representing the level required to access the
* function.
*/
AuthorizationLevel authLevel() default AuthorizationLevel.FUNCTION;
}