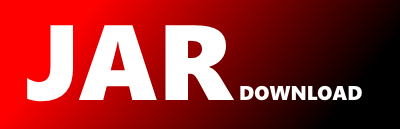
com.microsoft.azure.functions.annotation.CosmosDBTrigger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-functions-java-library Show documentation
Show all versions of azure-functions-java-library Show documentation
This package contains all Java interfaces and annotations to interact with Microsoft Azure functions runtime.
The newest version!
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*/
package com.microsoft.azure.functions.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
*
* Place this on a parameter whose value would come from CosmosDB, and causing the method to run
* when CosmosDB data is changed. The parameter type can be one of the following:
*
*
*
* - Some native Java types such as String
* - Nullable values using Optional<T>
* - Any POJO type
*
*
*
* The following example shows a Java function that is invoked when there are inserts or updates in
* the specified database and container.
*
*
*
* {@literal @}FunctionName("cosmosDBMonitor")
* public void cosmosDbLog(
* {@literal @}CosmosDBTrigger(name = "database",
* databaseName = "ToDoList",
* containerName = "Items",
* leaseContainerName = "leases",
* createLeaseContainerIfNotExists = true,
* connection = "AzureCosmosDBConnection")
* List<Map<String, String>> items,
* final ExecutionContext context
* ) {
* context.getLogger().info(items.size() + " item(s) is/are inserted.");
* if (!items.isEmpty()) {
* context.getLogger().info("The ID of the first item is: " + items.get(0).get("id"));
* }
* }
*
*
* @since 1.0.0
*/
@Retention(RetentionPolicy.RUNTIME)
@Target({ ElementType.PARAMETER })
public @interface CosmosDBTrigger {
/**
* The variable name used in function.json.
*
* @return The variable name used in function.json.
*/
String name();
/**
*
* Defines how Functions runtime should treat the parameter value. Possible values are:
*
*
* - "": get the value as a string, and try to deserialize to actual parameter type like
* POJO
* - string: always get the value as a string
* - binary: get the value as a binary data, and try to deserialize to actual parameter type
* byte[]
*
*
* @return The dataType which will be used by the Functions runtime.
*/
String dataType() default "";
/**
* Defines the database name of the CosmosDB to which to bind.
*
* @return The database name string.
*/
String databaseName();
/**
* Defines the container name of the CosmosDB to which to bind.
*
* @return The container name string.
*/
String containerName();
/**
* Defines Connection string for the service containing the lease container.
*
* @return Connection string for the lease container.
*/
String leaseConnectionStringSetting() default "";
/**
* Defines the lease container name of the CosmosDB to which to bind.
*
* @return The lease container name string.
*/
String leaseContainerName() default "";
/**
* Defines Name of the database containing the lease container.
*
* @return Name of the database for lease container.
*/
String leaseDatabaseName() default "";
/**
* Defines whether to create a new lease container if not exists.
*
* @return configuration whether to create a new lease container if not exists.
*/
boolean createLeaseContainerIfNotExists() default false;
/**
* defines the throughput of the created container.
*
* @return throughput
*/
int leasesContainerThroughput() default -1;
/**
* Defines a prefix to be used within a Leases container for this Trigger. Useful when sharing
* the same Lease container among multiple Triggers.
*
* @return LeaseContainerPrefix
*/
String leaseContainerPrefix() default "";
/**
* Customizes the amount of milliseconds between lease checkpoints. Default is always after a
* Function call.
*
* @return checkpointInterval
*/
int checkpointInterval() default -1;
/**
* Customizes the amount of documents between lease checkpoints. Default is always after a
* Function call.
*
* @return CheckpointDocumentCount
*/
int checkpointDocumentCount() default -1;
/**
* Customizes the delay in milliseconds in between polling a partition for new changes on the
* feed, after all current changes are drained. Default is 5000 (5 seconds).
*
* @return feedPollDelay
*/
int feedPollDelay() default 5000;
/**
* Defines the app setting name that contains the CosmosDB connection string.
*
* @return The app setting name of the connection string.
*/
String connection();
/**
* Customizes the renew interval in milliseconds for all leases for partitions currently held by
* the Trigger. Default is 17000 (17 seconds).
*
* @return renew interval in milliseconds for all leases
*/
int leaseRenewInterval() default 17000;
/**
* Customizes the interval in milliseconds to kick off a task to compute if partitions are
* distributed evenly among known host instances. Default is 13000 (13 seconds).
*
* @return interval in milliseconds
*/
int leaseAcquireInterval() default 13000;
/**
* Customizes the interval in milliseconds for which the lease is taken on a lease representing a
* partition. If the lease is not renewed within this interval, it will cause it to expire and
* ownership of the partition will move to another Trigger instance. Default is 60000 (60
* seconds).
*
* @return interval in milliseconds for which the lease is taken
*/
int leaseExpirationInterval() default 60000;
/**
* Customizes the maximum amount of items received in an invocation
*
* @return maximum amount of items received
*/
int maxItemsPerInvocation() default -1;
/**
* Gets or sets whether change feed in the Azure Cosmos DB service should start from beginning
* (true) or from current (false). By default it's start from current (false).
*
* @return Configuration whether change feed should start from beginning
*/
boolean startFromBeginning() default false;
/**
* Defines preferred locations (regions) for geo-replicated database accounts in the Azure Cosmos
* DB service. Values should be comma-separated. example, PreferredLocations = "East US,South
* Central US,North Europe"
*
* @return preferred locations (regions) for geo-replicated database accounts
*/
String preferredLocations() default "";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy