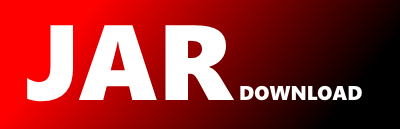
com.microsoft.azure.kusto.data.KustoResultMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kusto-data Show documentation
Show all versions of kusto-data Show documentation
Kusto client library for executing queries and retrieving data
package com.microsoft.azure.kusto.data;
import java.util.ArrayList;
import java.util.List;
import java.util.function.BiConsumer;
import java.util.function.Supplier;
/**
* A class for mapping Kusto results to a list of pojos
*
* @param
* pojo type returned by the mapping
*/
public class KustoResultMapper {
public static class Builder {
final List> queryResultColumns = new ArrayList<>();
final Supplier objConstructor;
public Builder(Supplier objConstructor) {
this.objConstructor = objConstructor;
}
/**
* Add a column by name. Using this function will cause the KustoResultMapper to lookup the column by name once per column per call to
* {@link KustoResultMapper#extractList(KustoResultSetTable)} or {@link KustoResultMapper#extractSingle(KustoResultSetTable)}
*
* @param
* Java type returned by the column (based on the KustoType parameter)
* @param type
* {@link KustoType} returned by the column
* @param name
* column name
* @param isNullable
* whether the column may contains null values
* @param setter
* function for setting a cell value into a pojo instance
* @return {@link Builder} of the provided type of the object being populated (determined by the object the setter belongs to)
*/
public Builder addColumn(KustoType type, String name, boolean isNullable, BiConsumer setter) {
this.queryResultColumns.add(KustoResultColumnPopulator.of(name, type, isNullable, setter));
return this;
}
/**
* Add a column by name and ordinal (column index). The ordinal value will be preferred for extracting values from the
* {@link KustoResultSetTable}.
*
* @param
* Java type returned by the column (based on the KustoType parameter)
* @param type
* {@link KustoType} returned by the column
* @param name
* column name
* @param ordinal
* index of the column in the
* @param isNullable
* whether the column may contains null values
* @param setter
* function for setting a cell value into a pojo instance
* @return {@link Builder} of the provided type of the object being populated (determined by the object the setter belongs to)
*/
public Builder addColumn(KustoType type, String name, int ordinal, boolean isNullable, BiConsumer setter) {
this.queryResultColumns.add(KustoResultColumnPopulator.of(name, ordinal, type, isNullable, setter));
return this;
}
/**
* Add a column by ordinal (column index).
*
* @param
* Java type returned by the column (based on the KustoType parameter)
* @param type
* {@link KustoType} returned by the column
* @param ordinal
* index of the column in the
* @param isNullable
* whether the column may contains null values
* @param setter
* function for setting a cell value into a pojo instance
* @return {@link Builder} of the provided type of the object being populated (determined by the object the setter belongs to)
*/
public Builder addColumn(KustoType type, int ordinal, boolean isNullable, BiConsumer setter) {
this.queryResultColumns.add(KustoResultColumnPopulator.of(ordinal, type, isNullable, setter));
return this;
}
public KustoResultMapper build() {
return new KustoResultMapper<>(this.queryResultColumns, this.objConstructor);
}
}
public static Builder newBuilder(Supplier objConstructor) {
return new Builder<>(objConstructor);
}
final List> columns;
final Supplier objConstructor;
private KustoResultMapper(List> columns, Supplier objConstructor) {
this.columns = columns;
this.objConstructor = objConstructor;
}
public R extractSingle(KustoResultSetTable resultSet) {
R ret = null;
if (resultSet.next()) {
ret = this.objConstructor.get();
for (KustoResultColumnPopulator col : this.columns) {
col.populateFrom(ret, resultSet);
}
}
return ret;
}
public List extractList(KustoResultSetTable resultSet) {
List ret = new ArrayList<>(resultSet.count());
int[] columnOrdinals = new int[this.columns.size()];
for (int i = 0; i < this.columns.size(); i++) {
columnOrdinals[i] = this.columns.get(i).columnIndexInResultSet(resultSet);
}
while (resultSet.next()) {
R resultObject = this.objConstructor.get();
for (int i = 0; i < this.columns.size(); i++) {
KustoResultColumnPopulator col = this.columns.get(i);
col.populateFrom(resultObject, resultSet, columnOrdinals[i]);
}
ret.add(resultObject);
}
return ret;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy