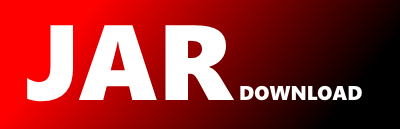
com.microsoft.applicationinsights.internal.schemav2.EventData Maven / Gradle / Ivy
/*
* ApplicationInsights-Java
* Copyright (c) Microsoft Corporation
* All rights reserved.
*
* MIT License
* Permission is hereby granted, free of charge, to any person obtaining a copy of this
* software and associated documentation files (the ""Software""), to deal in the Software
* without restriction, including without limitation the rights to use, copy, modify, merge,
* publish, distribute, sublicense, and/or sell copies of the Software, and to permit
* persons to whom the Software is furnished to do so, subject to the following conditions:
* The above copyright notice and this permission notice shall be included in all copies or
* substantial portions of the Software.
* THE SOFTWARE IS PROVIDED *AS IS*, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
* INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
* PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
* FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
* OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
* DEALINGS IN THE SOFTWARE.
*/
package com.microsoft.applicationinsights.internal.schemav2;
import java.io.IOException;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import com.microsoft.applicationinsights.telemetry.JsonTelemetryDataSerializer;
import com.google.common.base.Preconditions;
/**
* Data contract class EventData.
*/
public class EventData extends Domain {
/**
* Envelope Name for this telemetry.
*/
private static final String EVENT_ENVELOPE_NAME = "Microsoft.ApplicationInsights.Event";
/**
* Base Type for this telemetry.
*/
private static final String EVENT_BASE_TYPE = "EventData";
/**
* Backing field for property Ver.
*/
private int ver = 2;
/**
* Backing field for property Name.
*/
private String name;
/**
* Backing field for property Properties.
*/
private ConcurrentMap properties;
/**
* Backing field for property Measurements.
*/
private ConcurrentMap measurements;
/**
* Initializes a new instance of the class.
*/
public EventData() {
this.InitializeFields();
}
public int getVer() {
return this.ver;
}
public String getName() {
return this.name;
}
public void setName(String value) {
this.name = value;
}
public ConcurrentMap getProperties() {
if (this.properties == null) {
this.properties = new ConcurrentHashMap();
}
return this.properties;
}
public void setProperties(ConcurrentMap value) {
this.properties = value;
}
public ConcurrentMap getMeasurements() {
if (this.measurements == null) {
this.measurements = new ConcurrentHashMap();
}
return this.measurements;
}
public void setMeasurements(ConcurrentMap value) {
this.measurements = value;
}
protected void serializeContent(JsonTelemetryDataSerializer writer) throws IOException {
Preconditions.checkNotNull(writer, "writer must be a non-null value");
writer.write("ver", ver);
writer.write("name", name);
writer.write("properties", properties);
writer.write("measurements", measurements);
}
@Override
public String getEnvelopName() {
return EVENT_ENVELOPE_NAME;
}
@Override
public String getBaseTypeName() {
return EVENT_BASE_TYPE;
}
protected void InitializeFields() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy