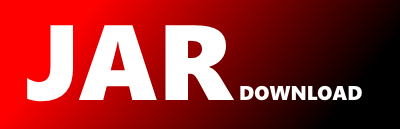
com.microsoft.azure.eventgrid.models.DeviceTwinInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-eventgrid Show documentation
Show all versions of azure-eventgrid Show documentation
This package contains Microsoft Azure EventGrid SDK.
/**
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for
* license information.
*
* Code generated by Microsoft (R) AutoRest Code Generator.
*/
package com.microsoft.azure.eventgrid.models;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Information about the device twin, which is the cloud representation of
* application device metadata.
*/
public class DeviceTwinInfo {
/**
* Authentication type used for this device: either SAS, SelfSigned, or
* CertificateAuthority.
*/
@JsonProperty(value = "authenticationType")
private String authenticationType;
/**
* Count of cloud to device messages sent to this device.
*/
@JsonProperty(value = "cloudToDeviceMessageCount")
private Double cloudToDeviceMessageCount;
/**
* Whether the device is connected or disconnected.
*/
@JsonProperty(value = "connectionState")
private String connectionState;
/**
* The unique identifier of the device twin.
*/
@JsonProperty(value = "deviceId")
private String deviceId;
/**
* A piece of information that describes the content of the device twin.
* Each etag is guaranteed to be unique per device twin.
*/
@JsonProperty(value = "etag")
private String etag;
/**
* The ISO8601 timestamp of the last activity.
*/
@JsonProperty(value = "lastActivityTime")
private String lastActivityTime;
/**
* Properties JSON element.
*/
@JsonProperty(value = "properties")
private DeviceTwinInfoProperties properties;
/**
* Whether the device twin is enabled or disabled.
*/
@JsonProperty(value = "status")
private String status;
/**
* The ISO8601 timestamp of the last device twin status update.
*/
@JsonProperty(value = "statusUpdateTime")
private String statusUpdateTime;
/**
* An integer that is incremented by one each time the device twin is
* updated.
*/
@JsonProperty(value = "version")
private Double version;
/**
* The thumbprint is a unique value for the x509 certificate, commonly used
* to find a particular certificate in a certificate store. The thumbprint
* is dynamically generated using the SHA1 algorithm, and does not
* physically exist in the certificate.
*/
@JsonProperty(value = "x509Thumbprint")
private DeviceTwinInfoX509Thumbprint x509Thumbprint;
/**
* Get authentication type used for this device: either SAS, SelfSigned, or CertificateAuthority.
*
* @return the authenticationType value
*/
public String authenticationType() {
return this.authenticationType;
}
/**
* Set authentication type used for this device: either SAS, SelfSigned, or CertificateAuthority.
*
* @param authenticationType the authenticationType value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
return this;
}
/**
* Get count of cloud to device messages sent to this device.
*
* @return the cloudToDeviceMessageCount value
*/
public Double cloudToDeviceMessageCount() {
return this.cloudToDeviceMessageCount;
}
/**
* Set count of cloud to device messages sent to this device.
*
* @param cloudToDeviceMessageCount the cloudToDeviceMessageCount value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withCloudToDeviceMessageCount(Double cloudToDeviceMessageCount) {
this.cloudToDeviceMessageCount = cloudToDeviceMessageCount;
return this;
}
/**
* Get whether the device is connected or disconnected.
*
* @return the connectionState value
*/
public String connectionState() {
return this.connectionState;
}
/**
* Set whether the device is connected or disconnected.
*
* @param connectionState the connectionState value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withConnectionState(String connectionState) {
this.connectionState = connectionState;
return this;
}
/**
* Get the unique identifier of the device twin.
*
* @return the deviceId value
*/
public String deviceId() {
return this.deviceId;
}
/**
* Set the unique identifier of the device twin.
*
* @param deviceId the deviceId value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withDeviceId(String deviceId) {
this.deviceId = deviceId;
return this;
}
/**
* Get a piece of information that describes the content of the device twin. Each etag is guaranteed to be unique per device twin.
*
* @return the etag value
*/
public String etag() {
return this.etag;
}
/**
* Set a piece of information that describes the content of the device twin. Each etag is guaranteed to be unique per device twin.
*
* @param etag the etag value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withEtag(String etag) {
this.etag = etag;
return this;
}
/**
* Get the ISO8601 timestamp of the last activity.
*
* @return the lastActivityTime value
*/
public String lastActivityTime() {
return this.lastActivityTime;
}
/**
* Set the ISO8601 timestamp of the last activity.
*
* @param lastActivityTime the lastActivityTime value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withLastActivityTime(String lastActivityTime) {
this.lastActivityTime = lastActivityTime;
return this;
}
/**
* Get properties JSON element.
*
* @return the properties value
*/
public DeviceTwinInfoProperties properties() {
return this.properties;
}
/**
* Set properties JSON element.
*
* @param properties the properties value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withProperties(DeviceTwinInfoProperties properties) {
this.properties = properties;
return this;
}
/**
* Get whether the device twin is enabled or disabled.
*
* @return the status value
*/
public String status() {
return this.status;
}
/**
* Set whether the device twin is enabled or disabled.
*
* @param status the status value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withStatus(String status) {
this.status = status;
return this;
}
/**
* Get the ISO8601 timestamp of the last device twin status update.
*
* @return the statusUpdateTime value
*/
public String statusUpdateTime() {
return this.statusUpdateTime;
}
/**
* Set the ISO8601 timestamp of the last device twin status update.
*
* @param statusUpdateTime the statusUpdateTime value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withStatusUpdateTime(String statusUpdateTime) {
this.statusUpdateTime = statusUpdateTime;
return this;
}
/**
* Get an integer that is incremented by one each time the device twin is updated.
*
* @return the version value
*/
public Double version() {
return this.version;
}
/**
* Set an integer that is incremented by one each time the device twin is updated.
*
* @param version the version value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withVersion(Double version) {
this.version = version;
return this;
}
/**
* Get the thumbprint is a unique value for the x509 certificate, commonly used to find a particular certificate in a certificate store. The thumbprint is dynamically generated using the SHA1 algorithm, and does not physically exist in the certificate.
*
* @return the x509Thumbprint value
*/
public DeviceTwinInfoX509Thumbprint x509Thumbprint() {
return this.x509Thumbprint;
}
/**
* Set the thumbprint is a unique value for the x509 certificate, commonly used to find a particular certificate in a certificate store. The thumbprint is dynamically generated using the SHA1 algorithm, and does not physically exist in the certificate.
*
* @param x509Thumbprint the x509Thumbprint value to set
* @return the DeviceTwinInfo object itself.
*/
public DeviceTwinInfo withX509Thumbprint(DeviceTwinInfoX509Thumbprint x509Thumbprint) {
this.x509Thumbprint = x509Thumbprint;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy