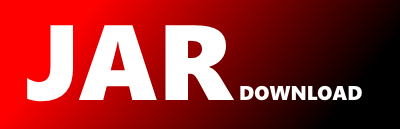
com.microsoft.azure.eventhubs.IEventHubClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-eventhubs Show documentation
Show all versions of azure-eventhubs Show documentation
Client library for talking to Microsoft Azure Event Hubs.
/*
* Copyright (c) Microsoft. All rights reserved.
* Licensed under the MIT license. See LICENSE file in the project root for full license information.
*/
package com.microsoft.azure.eventhubs;
import java.time.Instant;
import java.util.concurrent.CompletableFuture;
public interface IEventHubClient {
void sendSync(EventData data)
throws EventHubException;
CompletableFuture send(EventData data);
void sendSync(Iterable eventDatas)
throws EventHubException;
void sendSync(EventDataBatch eventDatas)
throws EventHubException;
CompletableFuture send(Iterable eventDatas);
CompletableFuture send(EventDataBatch eventDatas);
void sendSync(EventData eventData, String partitionKey)
throws EventHubException;
CompletableFuture send(EventData eventData, String partitionKey);
void sendSync(Iterable eventDatas, String partitionKey)
throws EventHubException;
CompletableFuture send(Iterable eventDatas, String partitionKey);
PartitionSender createPartitionSenderSync(String partitionId)
throws EventHubException, IllegalArgumentException;
CompletableFuture createPartitionSender(String partitionId)
throws EventHubException;
PartitionReceiver createReceiverSync(String consumerGroupName, String partitionId, String startingOffset)
throws EventHubException;
CompletableFuture createReceiver(String consumerGroupName, String partitionId, String startingOffset)
throws EventHubException;
PartitionReceiver createReceiverSync(String consumerGroupName, String partitionId, String startingOffset, boolean offsetInclusive)
throws EventHubException;
CompletableFuture createReceiver(String consumerGroupName, String partitionId, String startingOffset, boolean offsetInclusive)
throws EventHubException;
PartitionReceiver createReceiverSync(String consumerGroupName, String partitionId, Instant dateTime)
throws EventHubException;
CompletableFuture createReceiver(String consumerGroupName, String partitionId, Instant dateTime)
throws EventHubException;
PartitionReceiver createReceiverSync(String consumerGroupName, String partitionId, String startingOffset, ReceiverOptions receiverOptions)
throws EventHubException;
CompletableFuture createReceiver(String consumerGroupName, String partitionId, String startingOffset, ReceiverOptions receiverOptions)
throws EventHubException;
PartitionReceiver createReceiverSync(String consumerGroupName, String partitionId, String startingOffset, boolean offsetInclusive, ReceiverOptions receiverOptions)
throws EventHubException;
CompletableFuture createReceiver(String consumerGroupName, String partitionId, String startingOffset, boolean offsetInclusive, ReceiverOptions receiverOptions)
throws EventHubException;
PartitionReceiver createReceiverSync(String consumerGroupName, String partitionId, Instant dateTime, ReceiverOptions receiverOptions)
throws EventHubException;
CompletableFuture createReceiver(String consumerGroupName, String partitionId, Instant dateTime, ReceiverOptions receiverOptions)
throws EventHubException;
PartitionReceiver createEpochReceiverSync(String consumerGroupName, String partitionId, String startingOffset, long epoch)
throws EventHubException;
CompletableFuture createEpochReceiver(String consumerGroupName, String partitionId, String startingOffset, long epoch)
throws EventHubException;
PartitionReceiver createEpochReceiverSync(String consumerGroupName, String partitionId, String startingOffset, boolean offsetInclusive, long epoch)
throws EventHubException;
CompletableFuture createEpochReceiver(String consumerGroupName, String partitionId, String startingOffset, boolean offsetInclusive, long epoch)
throws EventHubException;
PartitionReceiver createEpochReceiverSync(String consumerGroupName, String partitionId, Instant dateTime, long epoch)
throws EventHubException;
CompletableFuture createEpochReceiver(String consumerGroupName, String partitionId, Instant dateTime, long epoch)
throws EventHubException;
PartitionReceiver createEpochReceiverSync(String consumerGroupName, String partitionId, String startingOffset, long epoch, ReceiverOptions receiverOptions)
throws EventHubException;
CompletableFuture createEpochReceiver(String consumerGroupName, String partitionId, String startingOffset, long epoch, ReceiverOptions receiverOptions)
throws EventHubException;
PartitionReceiver createEpochReceiverSync(String consumerGroupName, String partitionId, String startingOffset, boolean offsetInclusive, long epoch, ReceiverOptions receiverOptions)
throws EventHubException;
CompletableFuture createEpochReceiver(String consumerGroupName, String partitionId, String startingOffset, boolean offsetInclusive, long epoch, ReceiverOptions receiverOptions)
throws EventHubException;
PartitionReceiver createEpochReceiverSync(String consumerGroupName, String partitionId, Instant dateTime, long epoch, ReceiverOptions receiverOptions)
throws EventHubException;
CompletableFuture createEpochReceiver(String consumerGroupName, String partitionId, Instant dateTime, long epoch, ReceiverOptions receiverOptions)
throws EventHubException;
CompletableFuture onClose();
CompletableFuture getRuntimeInformation();
CompletableFuture getPartitionRuntimeInformation(String partitionId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy